How to change Floating Action Button Shape in Flutter
FloatingActionButton (FAB) is one of the important button widgets in Flutter. In this blog post, let’s learn how to change the shape of the Floating Action Button in Flutter.
The FloatingActionButton widget has many properties for style customization. Its shape property helps you to change the default shape of FAB. You can even add a border to the FAB using shape.
For example, if you want to change the FAB shape into a rectangle then you can use RoundedRectangleBorder class.
See the code snippet given below.
FloatingActionButton(
onPressed: () {},
shape: RoundedRectangleBorder(borderRadius: BorderRadius.circular(10)),
child: const Icon(Icons.add),
),
This will create a rounded rectangle Floating Action Button similar to the following output.
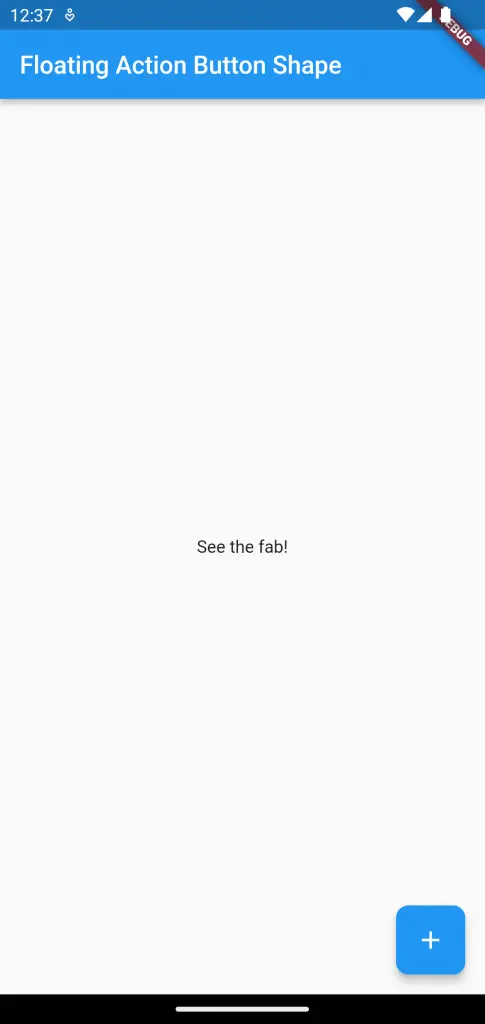
Following is the complete code.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Floating Action Button Shape'),
),
body: const Center(child: Text('See the fab!')),
floatingActionButton: FloatingActionButton(
onPressed: () {},
shape: RoundedRectangleBorder(borderRadius: BorderRadius.circular(10)),
child: const Icon(Icons.add),
),
);
}
}
That’s how you change the Floating Action Button shape in Flutter.