How to add Switch with Text in Flutter
A switch button is helpful to give input with any of two options by just toggling. In this blog post let’s learn how to add a Switch button with text or label in Flutter.
The Switch widget is used to create a switch button in Flutter. If you want to add a label beside the Switch then it’s better to use the SwitchListTile class.
The SwitchListTile widget is a mix of Switch and ListTile widgets. It has enough properties for customization. See the code snippet given below.
SwitchListTile(
tileColor: Colors.yellow[50],
activeColor: Colors.red,
title: const Text('Switch with text'),
value: selected,
onChanged: (bool? value) {
setState(() {
selected = value!;
});
},
)
You will get the following output.
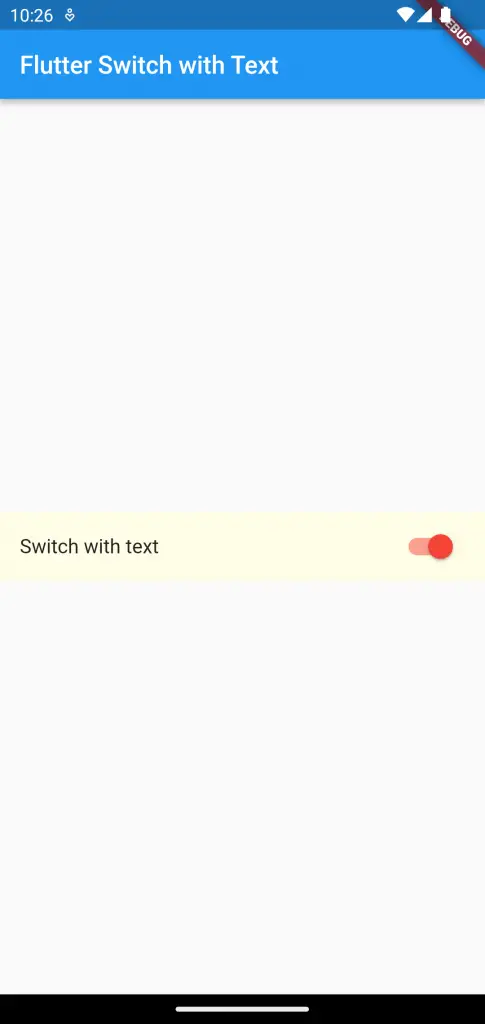
Following is the complete code.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Switch with Text'),
),
body: const MyStatefulWidget());
}
}
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({super.key});
@override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
bool selected = true;
@override
Widget build(BuildContext context) {
return Center(
child: SwitchListTile(
tileColor: Colors.yellow[50],
activeColor: Colors.red,
title: const Text('Switch with text'),
value: selected,
onChanged: (bool? value) {
setState(() {
selected = value!;
});
},
),
);
}
}
That’s how you add a Switch button with text in Flutter.