How to Add Padding to Column in Flutter
In this blog post, we’re diving deep into Flutter Column Padding. Padding plays a critical role in UI design. It can make your app look sleek or cluttered, depending on how you use it. Let’s get started!
The Need for Whole Column Padding
Sometimes, you’ll want padding around your entire column, not just individual widgets. Adding padding to the whole column sets the tone for your UI by providing a cushioned layout, which helps in separating your content from the edges of the screen.
Use the Padding Widget
The most straightforward way to add padding to an entire column is by wrapping the Column
widget with a Padding
widget. Here’s how:
const Padding(
padding: EdgeInsets.all(20.0),
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Text('First child'),
Text('Second child'),
],
),
)
This will add 20 units of padding on all sides of the column.
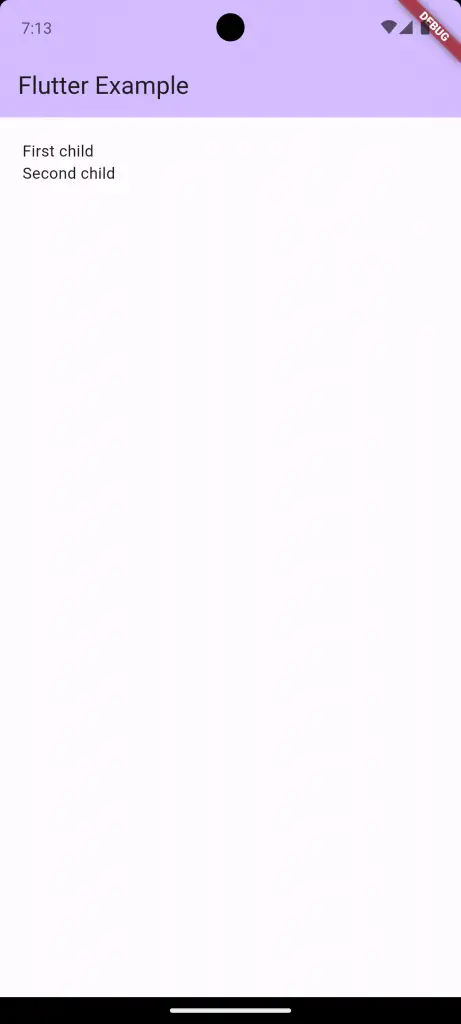
Use the Container Widget
Another approach is to use a Container
widget to wrap your Column
. The Container
widget offers a padding
property, too.
Container(
padding: const EdgeInsets.all(20.0),
child: const Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Text('First child'),
Text('Second child'),
],
),
)
The result is the same as using a Padding
widget, but using Container
gives you more styling options.
Best Practices for Column Padding
- Consistency is Key: Try to maintain the same padding values throughout your app for a cohesive look.
- Check Responsiveness: Always test the padding to make sure it looks good on various screen sizes.
Padding an entire column in Flutter is easy, yet crucial for any app’s aesthetic and user experience. You can use either the Padding
widget or a Container
to achieve this effect. Both are excellent options depending on your needs.