How to Add Margin to Column in Flutter
If you’ve been dabbling in Flutter, you know how crucial layout widgets like Column
are. They make vertical arrangement a breeze. But what if you want to add some space around your column?
In this blog post, we’re going to dive deep into adding margins to an Column
in Flutter.
Why Margin?
Before we get into the coding part, let’s talk about why margins are essential. Margins provide a buffer space around your Column
. This is especially useful when you don’t want your widgets to stick to the edges of the screen.
Add Margin Using Container Widget
One of the simplest ways to add a margin to your column is by wrapping it in a Container
widget.
Container(
margin: const EdgeInsets.all(20),
child: const Column(
children: [
Text("Hello"),
Text("World"),
],
),
)
This adds a uniform margin of 20 logical pixels around your column.
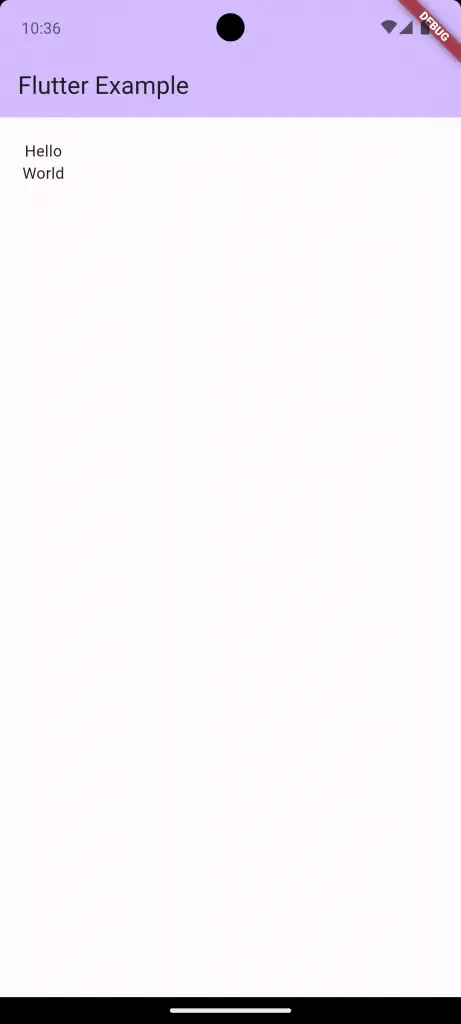
Following is the complete code for reference.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
colorScheme: ColorScheme.fromSeed(seedColor: Colors.deepPurple),
useMaterial3: true,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
backgroundColor: Theme.of(context).colorScheme.inversePrimary,
title: const Text(
'Flutter Example',
),
),
body: Container(
margin: const EdgeInsets.all(20),
child: const Column(
children: [
Text("Hello"),
Text("World"),
],
),
));
}
}
Customize with EdgeInsets
You might want different margins on different sides of your Column
. EdgeInsets
provides methods like only
to tailor it to your needs.
Container(
margin: const EdgeInsets.only(top: 20, left: 15),
child: const Column(
// Your widgets here
),
)
This code will only add a margin of 20 logical pixels to the top and 15 to the left.
A Real-world Example
Let’s see how to apply these concepts in a real-world scenario. Suppose you have a Column
that holds a profile picture, a username, and a bio.
Container(
margin: EdgeInsets.symmetric(horizontal: 20, vertical: 10),
child: Column(
children: [
CircleAvatar(
// Profile picture here
),
SizedBox(height: 10),
Text("Username"),
Text("Bio"),
],
),
)
In this example, we’ve used EdgeInsets.symmetric
to add horizontal and vertical margins easily.
Adding a margin to a Column
in Flutter is pretty straightforward. You can use a Container
, opt for a Padding
widget, or go all-in with EdgeInsets
for custom spacing. Each method has its perks, and the choice largely depends on your specific needs.