How to create Horizontal ListView in Flutter
The ListView widget helps to display a list of items efficiently in Flutter. By default, it scrolls in the vertical direction. In this Flutter tutorial, let’s learn how to add horizontal ListView easily.
The scrollDirection property of the ListView manages the direction. By making its value Axis.horizontal, you can scroll the ListView in the horizontal direction.
See the code snippet given below.
Container(
color: Colors.grey,
height: 100,
child: ListView.builder(
itemCount: data.length,
scrollDirection: Axis.horizontal,
itemBuilder: (BuildContext context, int index) {
return Container(
width: 100,
color: Colors.yellow,
margin: const EdgeInsets.all(8),
child: Center(child: Text(data[index])),
);
}))
As you see, the ListView is the child of the Container widget. This allows us to give a fixed height for the ListView.
You will get the following output.
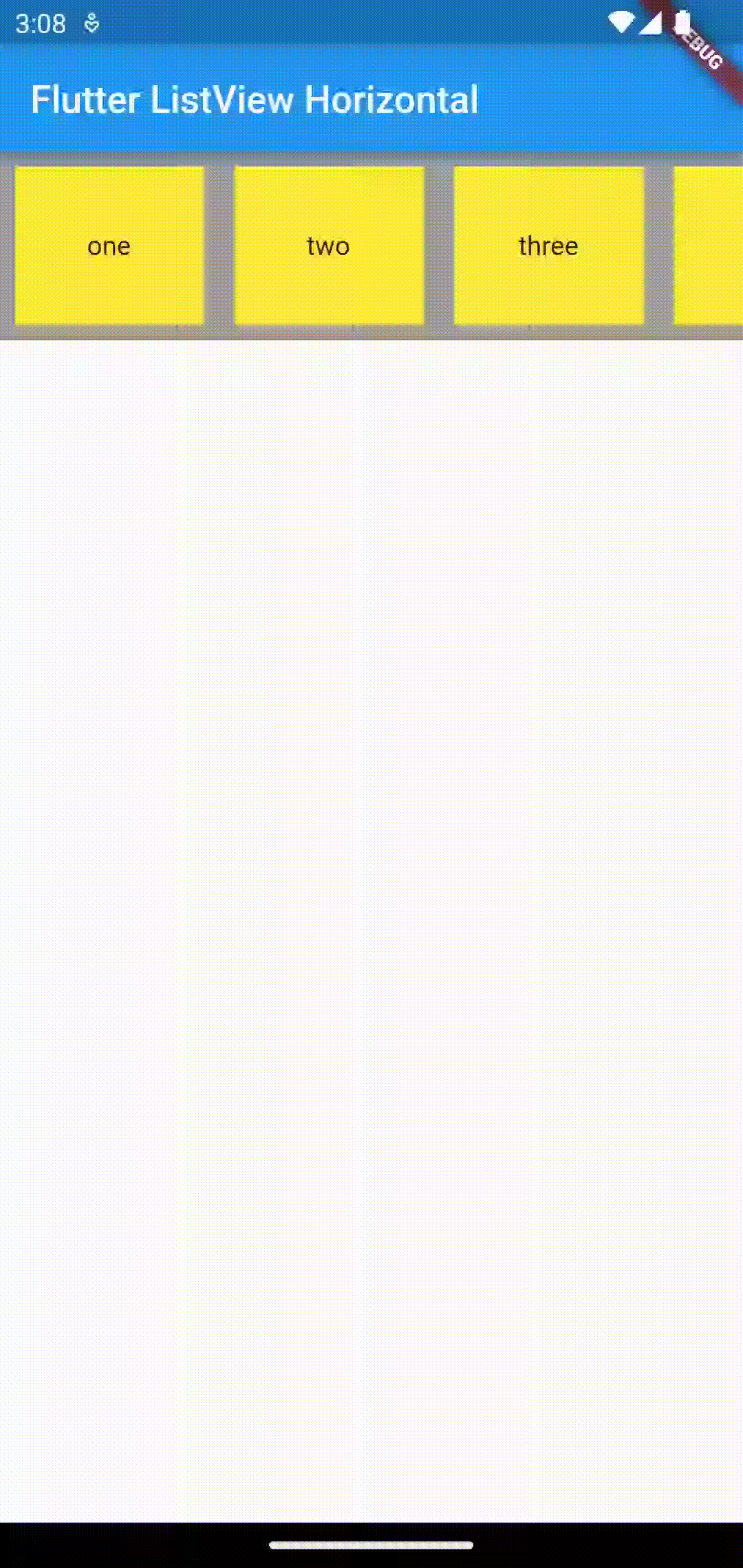
Following is the complete code.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
final data = [
"one",
"two",
"three",
"four",
"five",
"six",
"seven",
"eight",
"nine",
"ten"
];
MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text(
'Flutter ListView Horizontal',
),
),
body: Container(
color: Colors.grey,
height: 100,
child: ListView.builder(
itemCount: data.length,
scrollDirection: Axis.horizontal,
itemBuilder: (BuildContext context, int index) {
return Container(
width: 100,
color: Colors.yellow,
margin: const EdgeInsets.all(8),
child: Center(child: Text(data[index])),
);
})));
}
}
That’s how to make ListView scroll in the horizontal direction in Flutter.