How to Create Elevated Button with Icon and Text in Flutter
Many Flutter developers use ElevatedButton as their preferred button. In this blog post, let’s learn how to add an Elevated Button with Icon and text in Flutter.
You can add Elevated Button with an icon and text using ElevatedButton.icon constructor. Then you can make use of properties such as icon and label to add both icon and text.
ElevatedButton.icon(
icon: const Icon(
Icons.favorite,
color: Colors.pink,
size: 24.0,
),
label: const Text('Elevated Button'),
onPressed: () {},
)
The icon will be shown first and then the text follows. See the output given below.
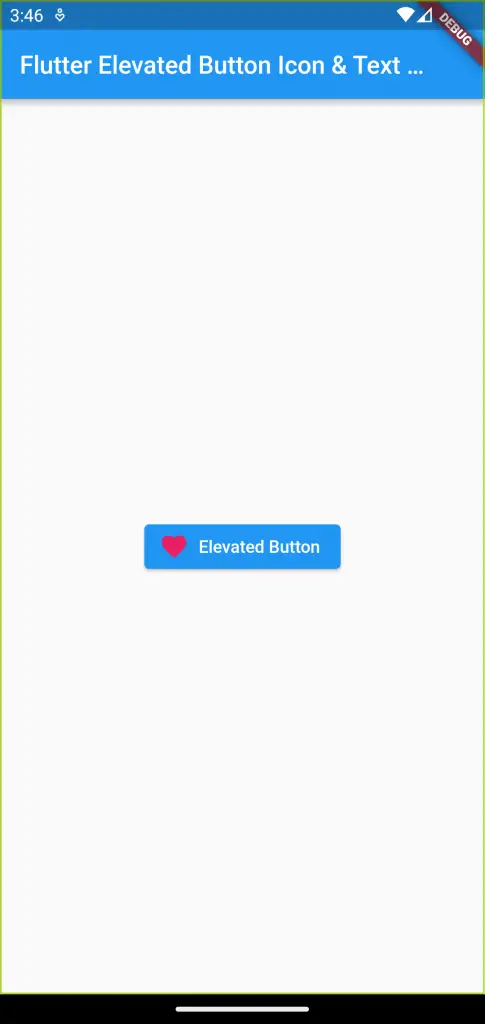
Following is the complete code for Flutter EleavatedButton with an icon example.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text(
'Flutter Elevated Button Icon & Text Example',
),
),
body: Center(
child: ElevatedButton.icon(
icon: const Icon(
Icons.favorite,
color: Colors.pink,
size: 24.0,
),
label: const Text('Elevated Button'),
onPressed: () {},
)));
}
}
That’s how you add an ElevatedButton widget with an icon and text in Flutter.
In the case of adding a button only with an icon, I suggest you to use the icon button instead of the elevated button.
I hope this Flutter tutorial to add ElevatedButton with icon and text is helpful for you.
how to add multiline text in elevatedbutton.icon?
because if i apply \n then padding removes automatically.