How to create a Card with Image and Text in Flutter
The cards are very useful to show information in mobile apps. In this blog post, let’s learn how to add a Card with image and text in Flutter.
You can create a card using the Card widget. Here, we use ListTile class as the child. It helps to accommodate an image and text easily in the Card.
See the code snippet given below.
Card(
margin: const EdgeInsets.all(20),
child: Column(
mainAxisSize: MainAxisSize.min,
children: <Widget>[
ListTile(
leading: Image.asset('images/sky.jpg'),
title: const Text('Demo Title'),
subtitle: const Text('This is a simple card in Flutter.'),
),
],
),
),
This will give you the following output.
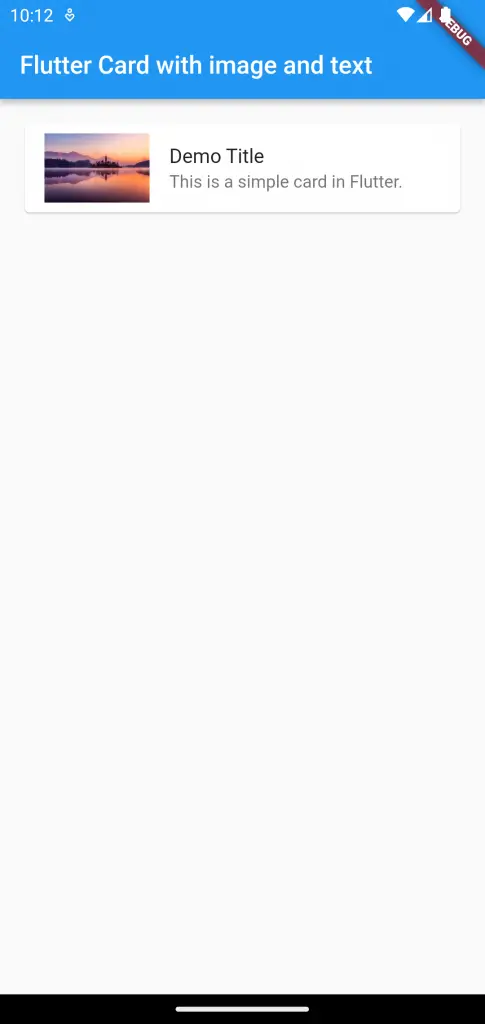
Following is the complete code.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
// colorSchemeSeed: const Color(0xff6750a4),
// useMaterial3: true,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Card with image and text'),
),
body: Card(
margin: const EdgeInsets.all(20),
child: Column(
mainAxisSize: MainAxisSize.min,
children: <Widget>[
ListTile(
leading: Image.asset('images/sky.jpg'),
title: const Text('Demo Title'),
subtitle: const Text('This is a simple card in Flutter.'),
),
],
),
),
);
}
}
That’s how you create a card with image and text in Flutter easily.