How to set Width and Height for Card in Flutter
The Card widget is a useful UI component to display information in Flutter. In this blog post, let’s learn how to set a fixed size for a Card in Flutter.
The Card widget doesn’t have properties for setting width and height. Hence we should wrap the card content with a SizedBox to define the size.
See the code snippet given below.
Card(
margin: const EdgeInsets.all(20),
child: SizedBox(
width: 250,
height: 100,
child: ListTile(
leading: Image.asset('images/sky.jpg'),
title: const Text('Demo Title'),
subtitle: const Text('This is a simple card in Flutter.'),
),
),
),
You will get an output of a Card with the given dimensions.
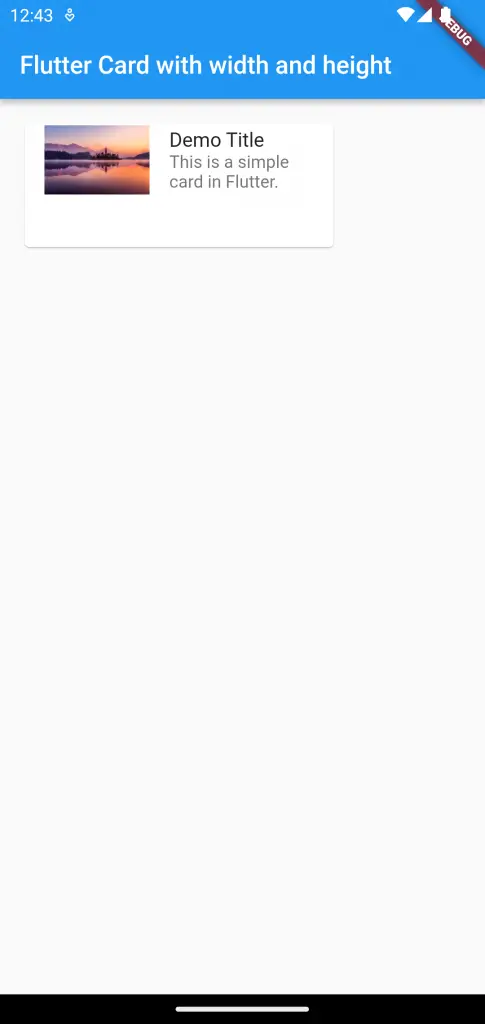
Following is the complete code.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
// colorSchemeSeed: const Color(0xff6750a4),
// useMaterial3: true,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Card with width and height'),
),
body: Card(
margin: const EdgeInsets.all(20),
child: SizedBox(
width: 250,
height: 100,
child: ListTile(
leading: Image.asset('images/sky.jpg'),
title: const Text('Demo Title'),
subtitle: const Text('This is a simple card in Flutter.'),
),
),
),
);
}
}
That’s how you set the height and width for the Card in Flutter.