How to add TextButton Border in Flutter
The TextButton widget helps to transform the text into a button easily. You can customize the TextButton in many ways. Let’s learn how to add a border to TextButton in this Flutter tutorial.
Borders help to give UI elements a distinct look. By leveraging TextButton.styleFrom() method you can style the button easily. Using the side property and BorderSide class you can show borders around the TextButton.
The code snippet is given below.
TextButton(
style: TextButton.styleFrom(
side: const BorderSide(width: 2, color: Colors.red),
padding: const EdgeInsets.all(10),
foregroundColor: Colors.red,
textStyle: const TextStyle(fontSize: 20)),
child: const Text('TextButton Border'),
onPressed: () {},
),
As you see, borders with the size of 2 and red color are applied. See the output given below.
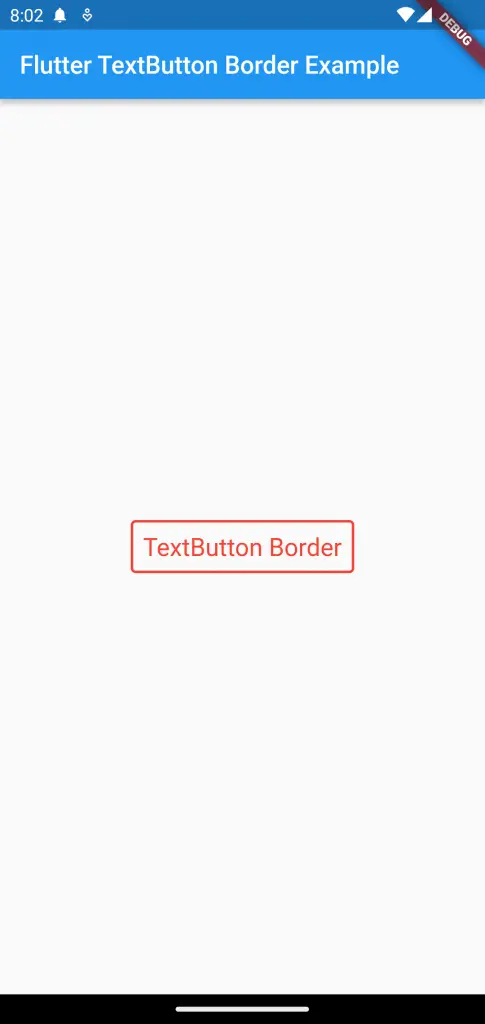
Following is the complete code for reference.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text(
'Flutter TextButton Border Example',
),
),
body: Center(
child: TextButton(
style: TextButton.styleFrom(
side: const BorderSide(width: 2, color: Colors.red),
padding: const EdgeInsets.all(10),
foregroundColor: Colors.red,
textStyle: const TextStyle(fontSize: 20)),
child: const Text('TextButton Border'),
onPressed: () {},
),
));
}
}
I hope this Flutter TextButton border tutorial is useful for you.