How to change ElevatedButton Icon Position to Right in Flutter
ElevatedButton is one of the most used button widgets in Flutter. In this Flutter tutorial, let’s learn how to change the position of the ElevatedButton icon from left to right.
When you create an ElevatedButton with an icon and text using ElevatedButton.icon, the icon appears at the left position.
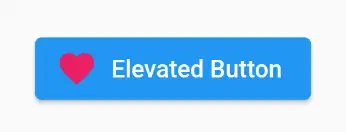
There are multiple ways to change the position of the icon to the right. One way is to use the Row widget as the child of the ElevatedButton. Then add Text and Icon in the desired order.
ElevatedButton(
onPressed: () {},
child: Row(mainAxisSize: MainAxisSize.min, children: const [
Text('Elevated Button'),
Icon(
Icons.favorite,
color: Colors.pink,
size: 24.0,
),
]),
)
You will get the following output.
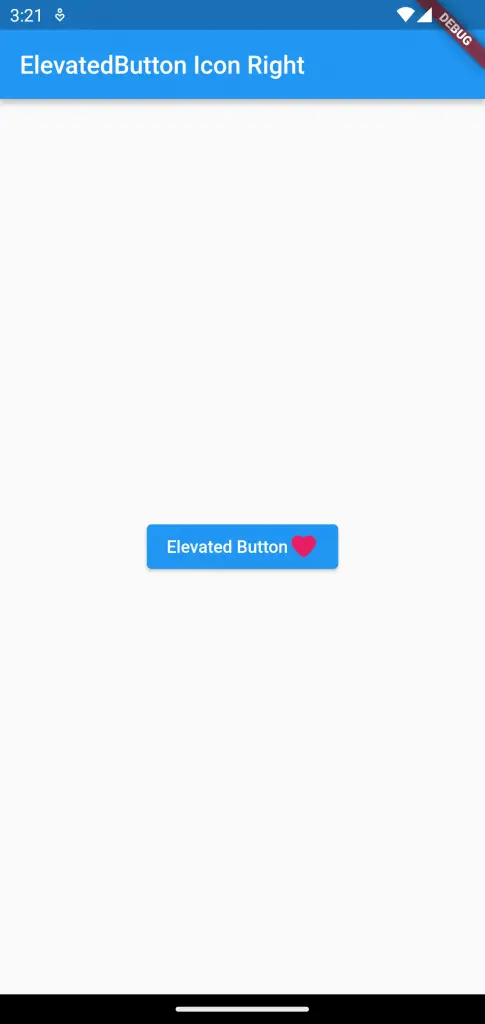
Following is the complete code.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('ElevatedButton Icon Right'),
),
body: Center(
child: ElevatedButton(
onPressed: () {},
child: Row(mainAxisSize: MainAxisSize.min, children: const [
Text('Elevated Button'),
Icon(
Icons.favorite,
color: Colors.pink,
size: 24.0,
),
]),
)));
}
}
An alternative solution to icon position is to use the Directionality widget. Make the ElevatedButton the child of the Directionality widget. Then make the text direction right to left. See the code snippet given below.
Directionality(
textDirection: TextDirection.rtl,
child: ElevatedButton.icon(
icon: const Icon(
Icons.favorite,
color: Colors.pink,
size: 24.0,
),
label: const Text('Elevated Button'),
onPressed: () {},
))
Then you will get the output as given below.
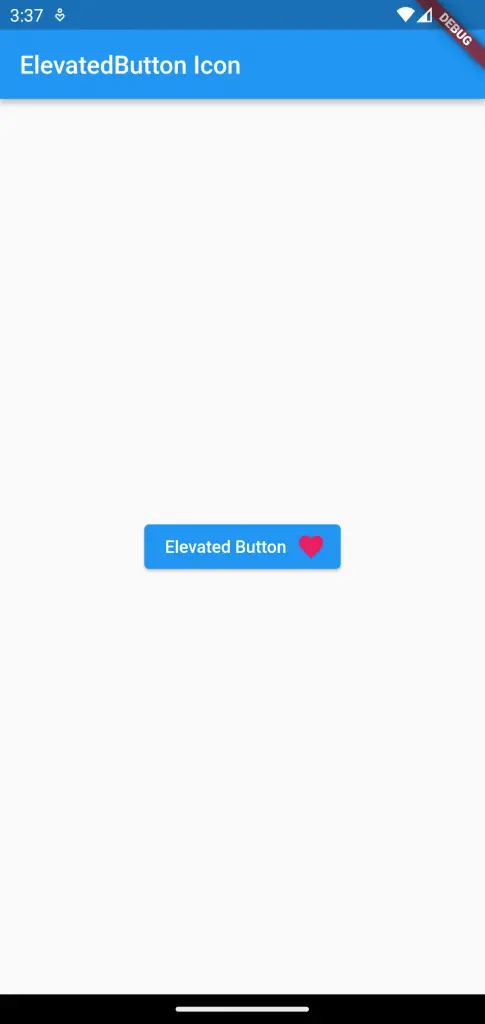
That’s how you change the position of the icon to right in the ElevatedButton.