How to Make Row Scrollable in Flutter
Flutter is a powerful tool for crafting beautiful UIs, and understanding how to make a scrollable Row
is essential for a seamless user experience, especially when dealing with horizontal lists of items that might extend beyond the screen width.
Let’s check the details of making a Row
widget scrollable in Flutter.
Why Make a Row Scrollable?
Sometimes, you have more items to show horizontally than what can fit on the screen. For instance, a list of images, tags, or small widgets like chips. In such cases, it’s user-friendly to have a scrollable Row
that allows users to swipe through the items.
Build a Scrollable Row
Let’s dive into the code and create a scrollable row from scratch.
Step 1: Set Up a Basic Row
Without scrollability, a basic Row
containing several Container
widgets might look like this:
Row(
children: <Widget>[
Container(width: 150, height: 100, color: Colors.red),
Container(width: 150, height: 100, color: Colors.green),
Container(width: 150, height: 100, color: Colors.blue),
],
)
This row will cause an overflow error if the combined width of the containers is greater than the screen width.
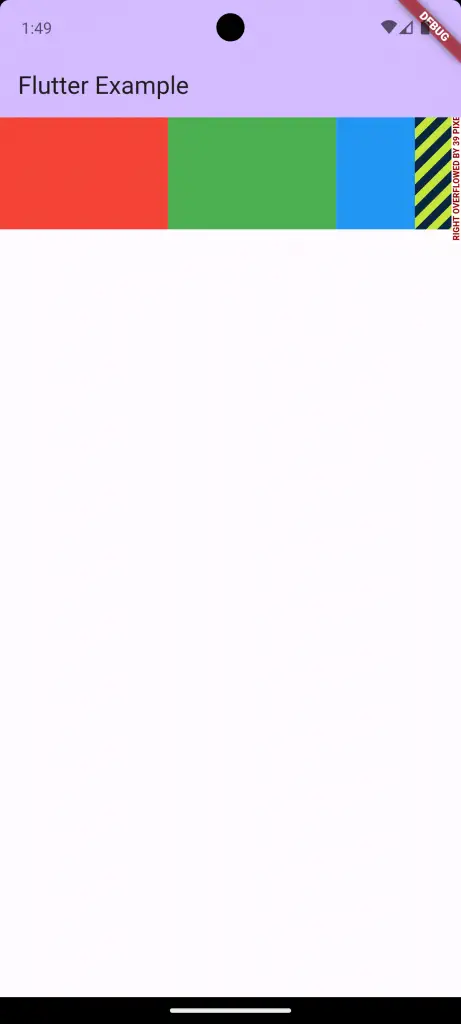
Step 2: Make the Row Scrollable
We wrap our Row
with a SingleChildScrollView
and specify the horizontal direction to enable scrolling:
SingleChildScrollView(
scrollDirection: Axis.horizontal,
child: Row(
children: <Widget>[
Container(width: 150, height: 100, color: Colors.red),
Container(width: 150, height: 100, color: Colors.green),
Container(width: 150, height: 100, color: Colors.blue),
],
),
)
Now, users can scroll through the containers horizontally.
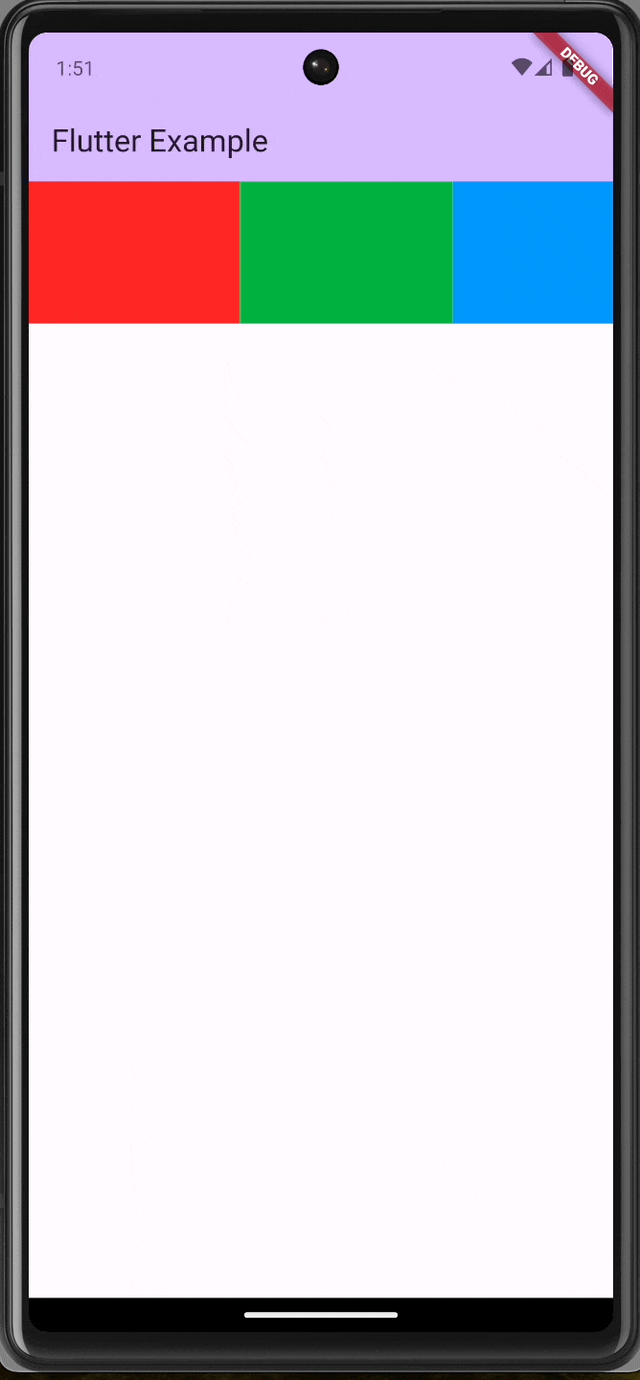
Considerations for a Better User Experience
- Performance: For a large number of containers, consider using a
ListView.builder
instead ofSingleChildScrollView
. It’s more efficient as it only renders the items that are currently visible on the screen. - Responsiveness: Always test your scrollable
Row
on various device sizes to ensure that the UI scales well.
A scrollable Row
in Flutter can significantly enhance your application’s interface, especially when dealing with horizontal lists. By following these steps, you can implement a smooth, scrollable row that accommodates any number of containers, ensuring a clean and user-friendly design.
One Comment