How to change Order of Items in a ListView using Drag and Drop in Flutter
I already have a couple of Flutter tutorials on the ListView widget. Here, I am going to demonstrate a Flutter tutorial where the order of items of a ListView can be changed. What this really means is a user can change the order of items by just dragging and dropping.
Undoubtedly, ListView is one of the most commonly used widgets in Flutter. Here, we are going to use another widget named ReorderableListView. The ReorderableListView widget is very appropriate for views with a small number of children.
If you have a large list of items then you should prefer ReorderableListView.builder constructor.
All children of ReorderableListView must have a key. Following is a Flutter example where the order of children can be changed by dragging to the up or down position. The onReorder property is used to define action after reordering. Usually, we have to update data on reordering.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter ListView Example'),
),
body: const MyStatefulWidget());
}
}
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({super.key});
@override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
var data = [
"Hey",
"What's",
"Up",
"Dude",
"This",
"is",
"a",
"Flutter",
"example",
"got",
"it"
];
@override
Widget build(BuildContext context) {
return ReorderableListView(
onReorder: (oldIndex, newIndex) {
setState(() {
_updateItems(oldIndex, newIndex);
});
},
children: [
for (final item in data)
Container(
key: ValueKey(item),
height: 150,
color: Colors.white,
child:
Center(child: Text(item, style: const TextStyle(fontSize: 30))),
)
],
);
}
void _updateItems(int oldIndex, int newIndex) {
if (newIndex > oldIndex) {
newIndex -= 1;
}
final item = data.removeAt(oldIndex);
data.insert(newIndex, item);
}
}
Following is the output of the above Flutter example.
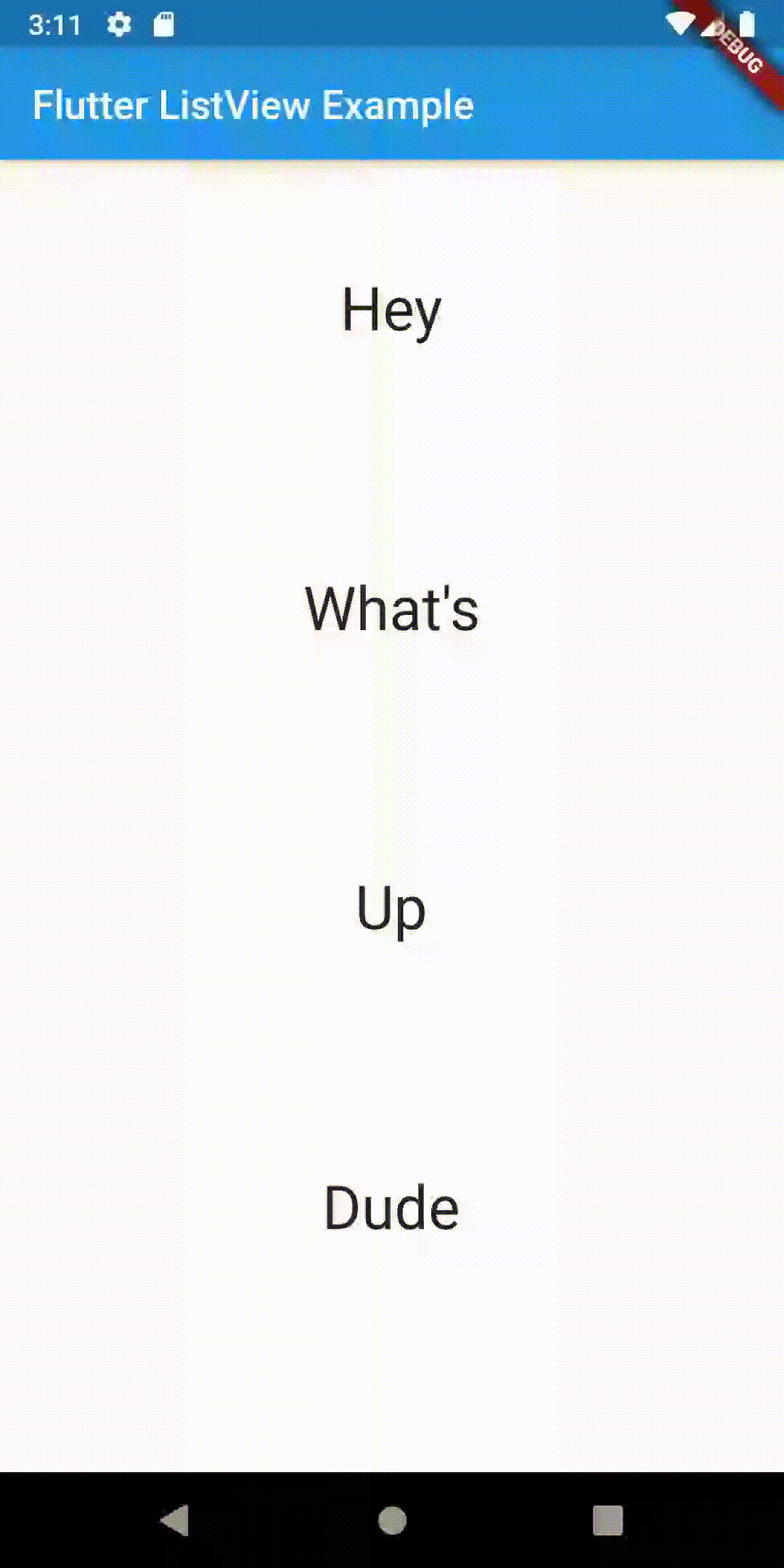
That’s how you add drag and drop feature to re-order items in Flutter ListView.