How to add Border to Card in Flutter
A Card widget is generally used to show information to users. Hence, it’s important to make the Card looks neat and beautiful. In this blog post, let’s learn how to add a Flutter Card with a border.
You can add borders to the Card widget using the shape property. Making use of RoundedRectangleBorder class helps you to customize border width, border color, border radius, etc.
See the code snippet given below.
Card(
margin: const EdgeInsets.all(20),
elevation: 5,
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(20),
side: const BorderSide(width: 1, color: Colors.red)),
color: Colors.yellow[100],
child: Column(
mainAxisSize: MainAxisSize.min,
children: const <Widget>[
ListTile(
title: Text('Demo Title'),
subtitle: Text('This is a simple card in Flutter.'),
),
],
),
)
And you will get the output with red color borders.
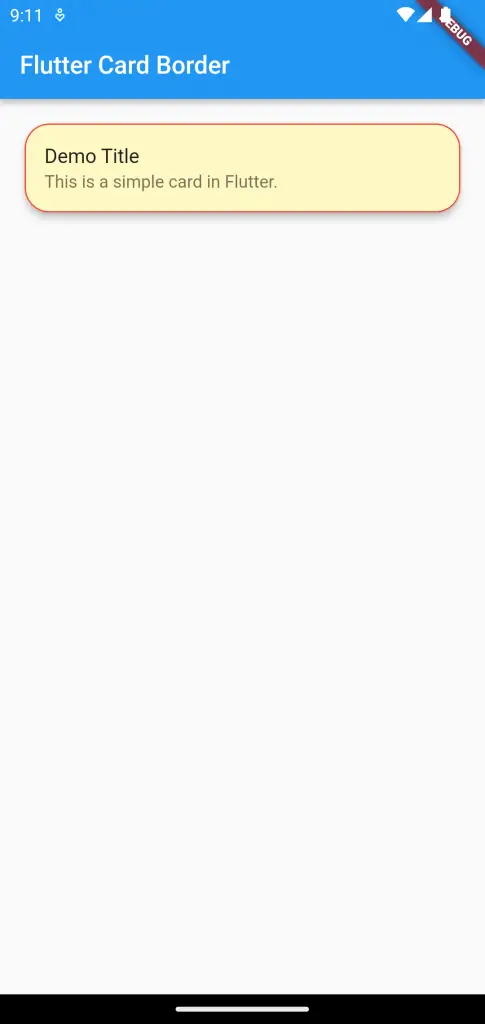
Following is the complete code.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
// colorSchemeSeed: const Color(0xff6750a4),
// useMaterial3: true,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Card Border'),
),
body: Card(
margin: const EdgeInsets.all(20),
elevation: 5,
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(20),
side: const BorderSide(width: 1, color: Colors.red)),
color: Colors.yellow[100],
child: Column(
mainAxisSize: MainAxisSize.min,
children: const <Widget>[
ListTile(
title: Text('Demo Title'),
subtitle: Text('This is a simple card in Flutter.'),
),
],
),
),
);
}
}
I hope this tutorial to add borders to the Card in Flutter is helpful for you.