How to make Card Clickable in Flutter
The Card widget is one of the most used widgets in Flutter. Sometimes, we may want to make the whole card clickable. In this Flutter tutorial, let’s learn how to make a Card clickable.
Making a Card clickable is not a difficult task. All you need to use is the InkWell widget and nest your widgets in it. Then make the InkWell the child of the Card.
See the code snippet given below.
Card(
margin: const EdgeInsets.all(20),
child: InkWell(
onTap: () {},
splashColor: Colors.blue,
child: Column(
mainAxisSize: MainAxisSize.min,
children: const <Widget>[
ListTile(
title: Text('Demo Title'),
subtitle: Text('This is a simple card in Flutter.'),
),
],
),
),
),
Then you will get the following output.
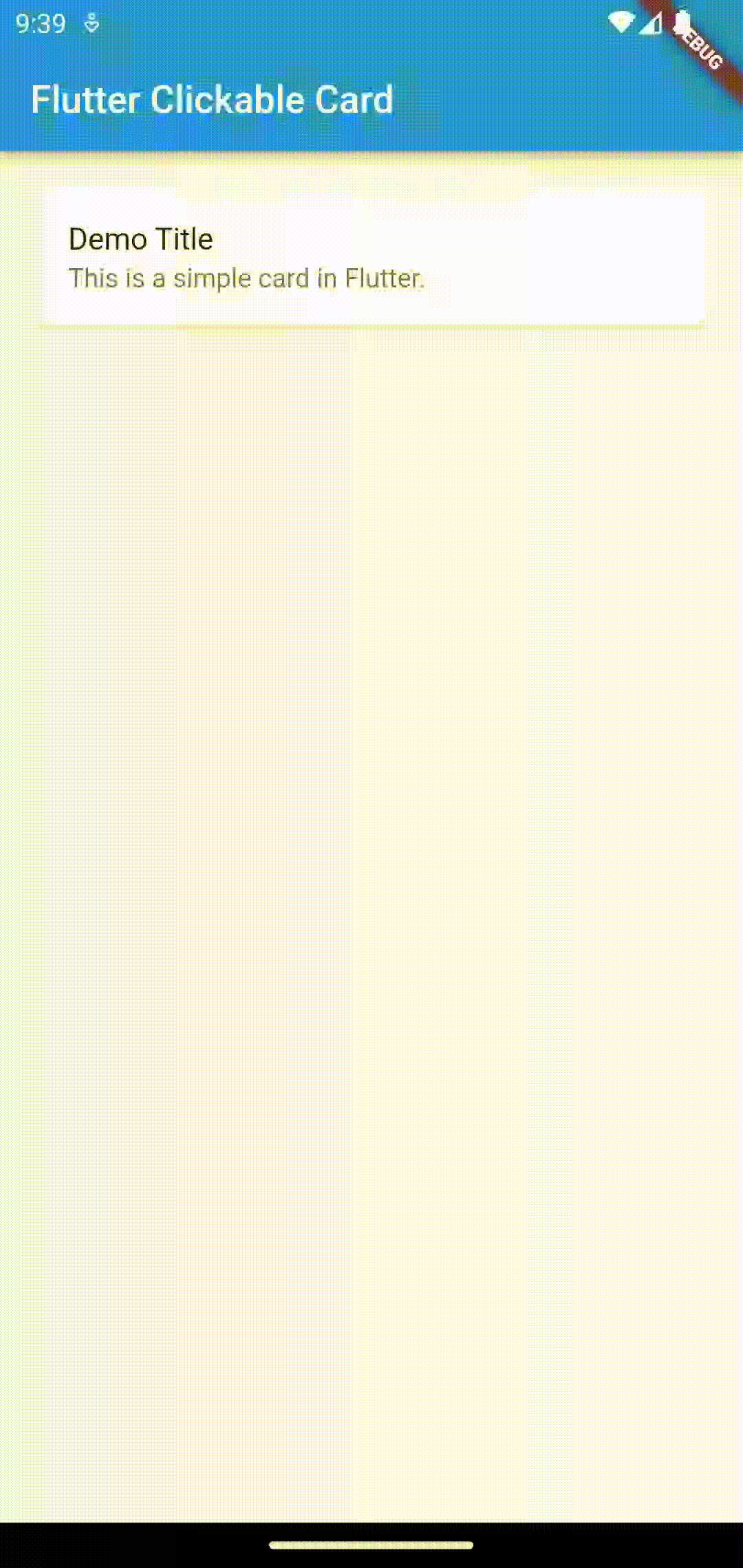
Following is the complete code.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
// colorSchemeSeed: const Color(0xff6750a4),
// useMaterial3: true,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Clickable Card'),
),
body: Card(
margin: const EdgeInsets.all(20),
child: InkWell(
onTap: () {},
splashColor: Colors.blue,
child: Column(
mainAxisSize: MainAxisSize.min,
children: const <Widget>[
ListTile(
title: Text('Demo Title'),
subtitle: Text('This is a simple card in Flutter.'),
),
],
),
),
),
);
}
}
That’s how you make the Card widget clickable in Flutter.