How to Create Expandable Widget in Flutter
In some situations, we may want to create widgets that are expandable to fill the unwanted space that props between other widgets. In this blog post, let’s check how to create an expandable widget in Flutter.
You can make use of the Expanded widget for this purpose. It accepts properties such as flex so that the expansion of widgets can be made controllable.
In the following flutter example, we have three container widgets which are children of the Row widget. And the last container widget is wrapped with an expandable widget.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text(
'Flutter Row Example With Expandable',
),
),
body: Center(
child: Row(
children: <Widget>[
Container(
color: Colors.red,
height: 100,
width: 100,
),
Container(
color: Colors.yellow,
height: 100,
width: 100,
),
Expanded(
child: Container(
color: Colors.blue,
height: 100,
width: 100,
),
)
],
),
),
);
}
}
And you can see how the third container behaved by looking into the following output.
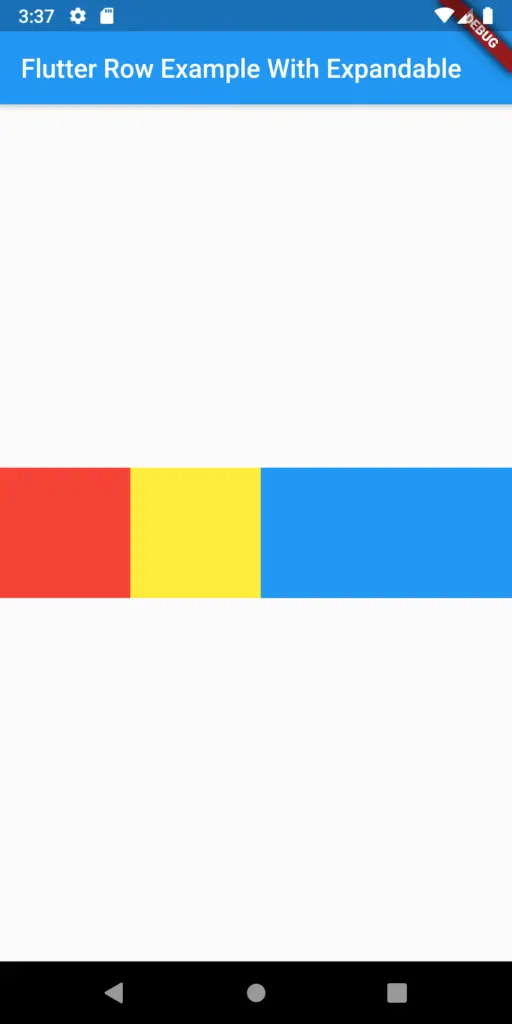
Expanded Widget using Flex
The behavior changes when we use the flex property with the Expanded widget.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text(
'Flutter Row Example With Expandable',
),
),
body: Center(
child: Row(
children: <Widget>[
Expanded(
flex: 2,
child: Container(
color: Colors.red,
height: 100,
width: 100,
),
),
Expanded(
flex: 1,
child: Container(
color: Colors.yellow,
height: 100,
width: 100,
),
),
Expanded(
flex: 3,
child: Container(
color: Colors.blue,
height: 100,
width: 100,
),
)
],
),
),
);
}
}
And following is the output.
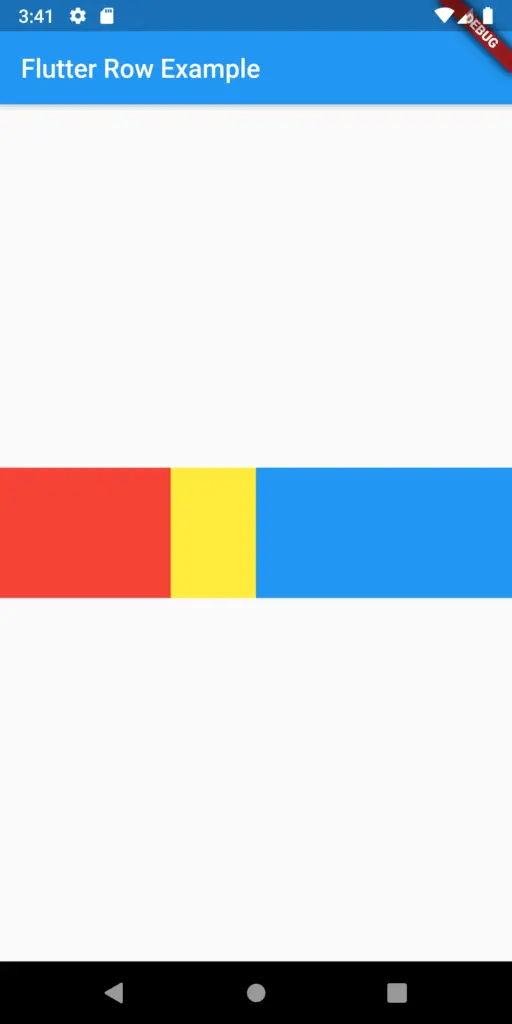
Thank you for reading!