How to change OutlinedButton Text Color in Flutter
Buttons are one of the most important UI components in mobile app design. Hence, choosing the right colors for buttons is necessary. In this short Flutter tutorial, let’s learn how to change the OutlinedButton text color.
The OutlinedButton.styleFrom has various properties to change the style. The backgroundColor property changes the background color whereas the foregroundColor property changes the color of its child such as Text, Icon, etc.
See the code snippet given below.
OutlinedButton(
onPressed: () {},
style: OutlinedButton.styleFrom(
backgroundColor: Colors.red, foregroundColor: Colors.white),
child: const Text('Outlined Button'),
)
You will get the output with white color Text and red color background. Alternatively, you can also change the text color with the TextStyle class.
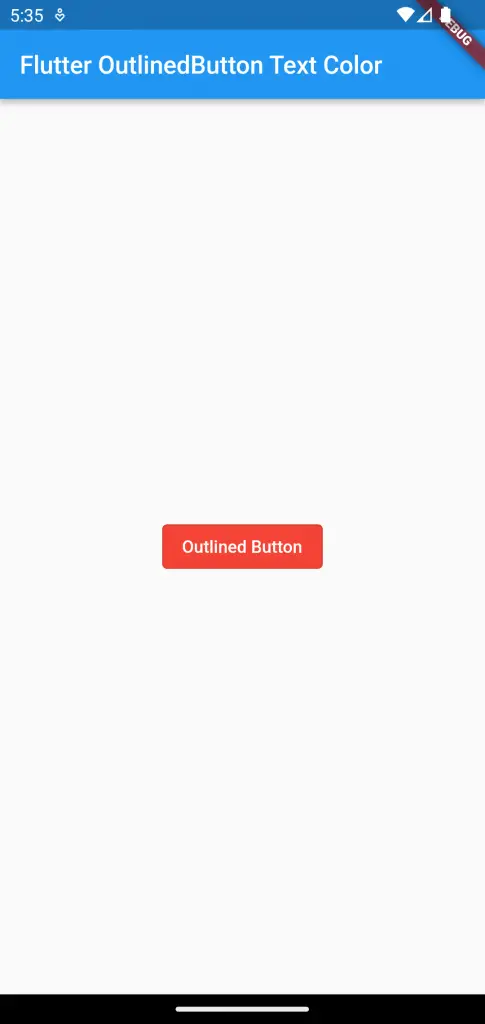
Following is the complete code for reference.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter OutlinedButton Text Color'),
),
body: Center(
child: OutlinedButton(
onPressed: () {},
style: OutlinedButton.styleFrom(
backgroundColor: Colors.red, foregroundColor: Colors.white),
child: const Text('Outlined Button'),
)));
}
}
I hope this Flutter tutorial to change OutlinedButton to change text color is helpful for you.