How to Convert Base64 to PDF in Flutter
Base64 is a popular encoding format for representing binary data as text, and it’s widely used for encoding and decoding data in various applications. In this blog post, let’s learn how to convert base64 to PDF in Flutter.
In this tutorial, we use a sample base64 string and convert it into PDF. Then we open the pdf file using the pdf viewer.
Here, we use two packages, path_provider, and open_filex. Make sure you have installed them into your Flutter project.
The following function will convert the base64 to pdf.
createPdf() async {
var base64 =
'JVBERi0xLjQKJcOkw7zDtsOfCjIgMCB......';
var bytes = base64Decode(base64);
final output = await getTemporaryDirectory();
final file = File("${output.path}/example.pdf");
await file.writeAsBytes(bytes.buffer.asUint8List());
debugPrint("${output.path}/example.pdf");
await OpenFilex.open("${output.path}/example.pdf");
}
This is an asynchronous function that is used to convert a Base64 string representation of a PDF file into an actual PDF file in Flutter.
It initializes a Base64 string base64 that represents a PDF file. The base64Decode() function is used to convert the base64 string into a list of bytes, which is stored in the bytes variable.
The getTemporaryDirectory() function from the path provider package is used to get the path of the temporary directory on the device where the PDF file will be stored.
The File class is used to create a new file in the temporary directory with the name example.pdf. The writeAsBytes() method is used to write the bytes to the file.
The OpenFilex.open() function is used to open the newly created PDF file using the specified path.
You will get the following output.
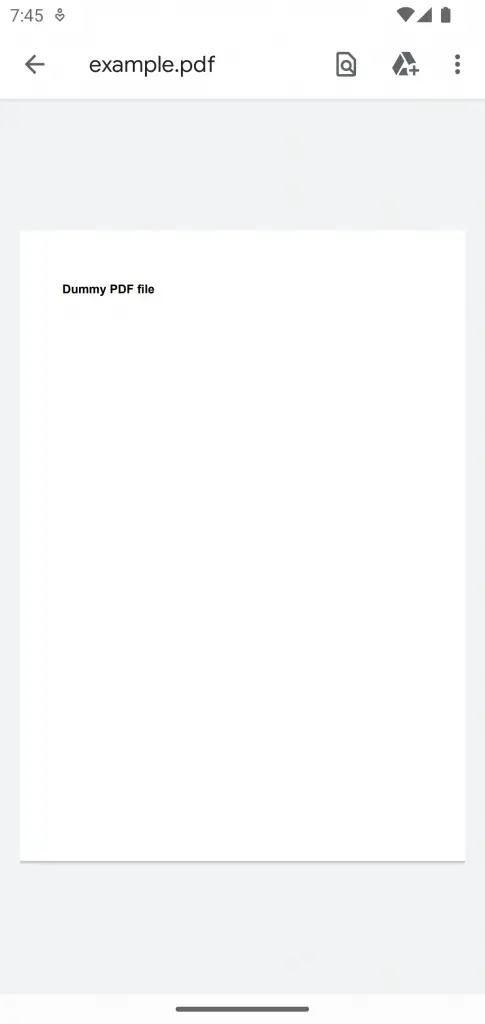
Following is the complete code.
import 'dart:convert';
import 'package:flutter/material.dart';
import 'package:open_filex/open_filex.dart';
import 'dart:io';
import 'package:path_provider/path_provider.dart';
void main() => runApp(const MyApp());
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
useMaterial3: true,
),
home: const MyStatefulWidget());
}
}
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({super.key});
@override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
createPdf() async {
var base64 =
'JVBERi0xLjQKJcOkw7zDtsOfCjIgMCBv...................4vis65K9R4cmVmCjEyNzg3CiUlRU9GCg==';
var bytes = base64Decode(base64);
final output = await getTemporaryDirectory();
final file = File("${output.path}/example.pdf");
await file.writeAsBytes(bytes.buffer.asUint8List());
debugPrint("${output.path}/example.pdf");
await OpenFilex.open("${output.path}/example.pdf");
}
@override
void initState() {
super.initState();
createPdf();
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter PDF Example'),
),
body: const Text('wait...'),
);
}
}
That’s how you show PDF from base64 in Flutter.