How to set Background Image for Card in Flutter
The Card is one of the useful UI components in the mobile app. It helps to display information in a convenient way. In this blog post, let’s learn how to set a Card background image in Flutter.
The Card widget doesn’t have a property for this. Hence, we should add a widget that supports setting the background image as the child of the Card. We use the Container widget and the BoxDecoration class.
See the following code snippet.
Card(
margin: const EdgeInsets.all(10),
shape: RoundedRectangleBorder(borderRadius: BorderRadius.circular(10)),
clipBehavior: Clip.antiAliasWithSaveLayer,
elevation: 5,
child: Container(
decoration: const BoxDecoration(
image: DecorationImage(
image: AssetImage("images/sky.jpg"),
fit: BoxFit.cover,
alignment: Alignment.topCenter,
),
),
child: const ListTile(
title: Text('Demo Title'),
subtitle: Text('This is a simple card in Flutter.'),
),
),
),
You will get the following output.
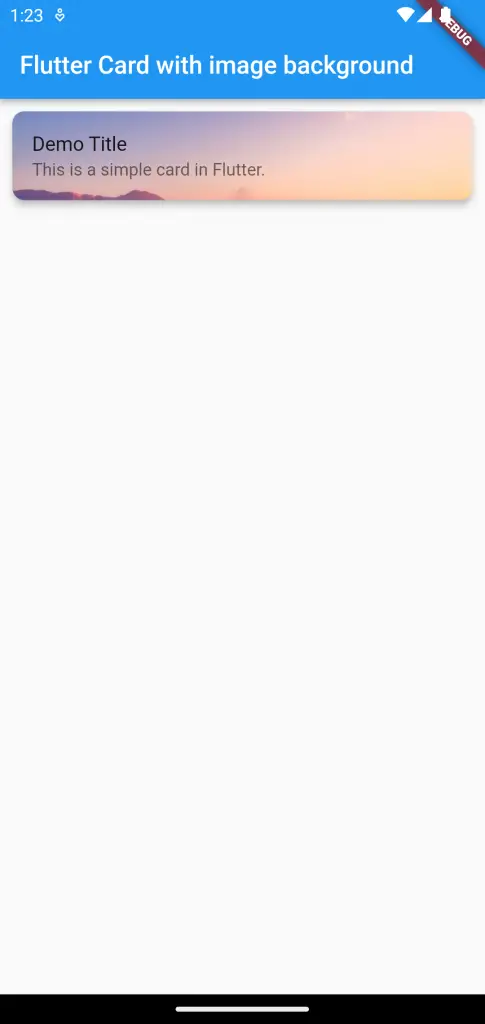
If you want to add borders then see this tutorial.
Following is the complete code.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
// colorSchemeSeed: const Color(0xff6750a4),
// useMaterial3: true,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Card with image background'),
),
body: Card(
margin: const EdgeInsets.all(10),
shape: RoundedRectangleBorder(borderRadius: BorderRadius.circular(10)),
clipBehavior: Clip.antiAliasWithSaveLayer,
elevation: 5,
child: Container(
decoration: const BoxDecoration(
image: DecorationImage(
image: AssetImage("images/sky.jpg"),
fit: BoxFit.cover,
alignment: Alignment.topCenter,
),
),
child: const ListTile(
title: Text('Demo Title'),
subtitle: Text('This is a simple card in Flutter.'),
),
),
),
);
}
}
That’s how you set a background image for the Card in Flutter.
If you want to set a gradient background then see this tutorial.