How to Create TextField for Password in Flutter
The importance of passwords is inexplainable. That’s the reason when entering the password the text should not be visible for better safety and security. In this Flutter tutorial, let’s see how to create a Text Input to enter a password in Flutter.
I already have a blog post on how to create a Text Input in Flutter. As I mentioned in that blog post, we use the TextField widget to create inputs in Flutter.
The TextField widget has so many useful properties. The property obscureText can be used to hide the text which is being edited. You just need to set the obscureText true.
See the code snippet given below.
TextField(
controller: _controller,
obscureText: true,
onSubmitted: (String value) {
debugPrint(value);
},
),
And you will get the following output.
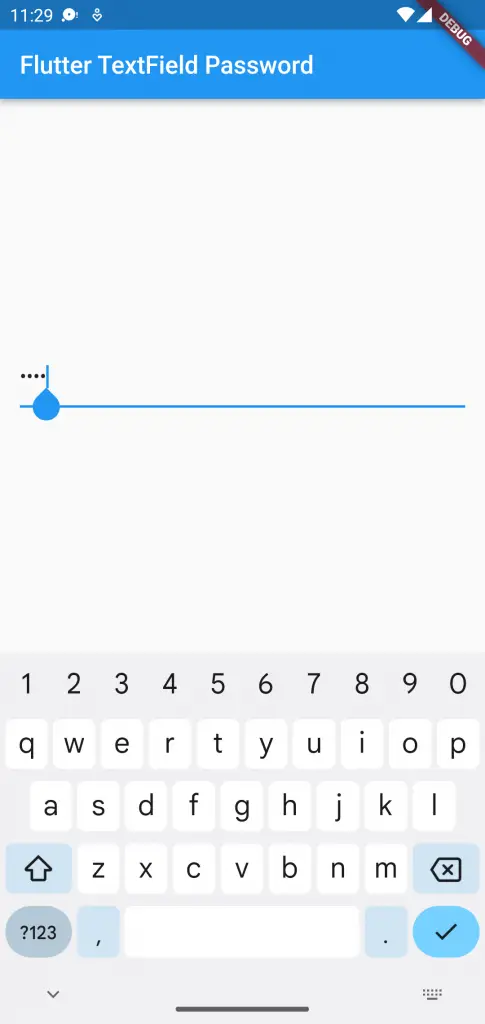
Following is the complete example of Flutter TextField for password entry.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter TextField Password'),
),
body: const MyStatefulWidget());
}
}
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({super.key});
@override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
late TextEditingController _controller;
@override
void initState() {
super.initState();
_controller = TextEditingController();
}
@override
void dispose() {
_controller.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Center(
child: Padding(
padding: const EdgeInsets.all(16.0),
child: Center(
child: TextField(
controller: _controller,
obscureText: true,
onSubmitted: (String value) {
debugPrint(value);
},
),
),
));
}
}
I hope this Flutter TextField tutorial to hide password is useful for you.
Thank you for reading!