How to Add Separators to Flutter Column
When building apps, one of the most basic yet essential tasks is organizing your UI elements well. That’s where separators come into play. Separators can help make your app’s layout more readable and visually appealing.
In this blog post, we’ll focus on how to add separators to a Flutter Column
.
The Importance of Separators
First things first, why even bother with separators? The answer is simple: they enhance user experience. When you have a list of items, separators can help break it down into digestible pieces, making it easier for the user to interact with your app.
Divider Widget
The easiest way to add a separator to your Column
is by incorporating the Divider
widget.
const Column(
children: [
Text('First Child'),
Divider(),
Text('Second Child'),
Divider(),
Text('Third Child'),
],
)
Here, we use the Divider
widget between Text
widgets in a Column
. The Divider
creates a horizontal line, acting as a separator between the elements. This is one of the simplest yet most effective ways to add separators in a Column
.
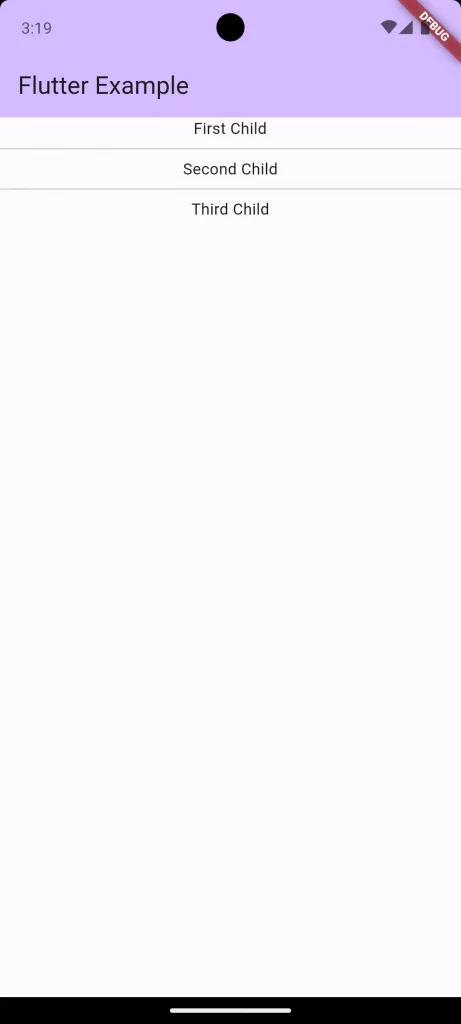
Following is the complete code for reference.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
colorScheme: ColorScheme.fromSeed(seedColor: Colors.deepPurple),
useMaterial3: true,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
backgroundColor: Theme.of(context).colorScheme.inversePrimary,
title: const Text(
'Flutter Example',
),
),
body: const Column(
children: [
Text('First Child'),
Divider(),
Text('Second Child'),
Divider(),
Text('Third Child'),
],
));
}
}
Separators are a small but significant part of your Flutter app’s UI. They help improve readability and the overall user experience. The Divider
widget is a straightforward and effective way to add these separators in a Column
. So the next time you find your UI looking a bit cluttered, consider using separators to tidy things up.