How to change AlertDialog Border Radius in Flutter
Tweaking border-radius helps us to create rounded corners. UI elements with rounded corners always look beautiful. In this Flutter tutorial, let’s learn how to change the border radius of AlertDialog.
Following is the default border radius of AlertDialog in Flutter.
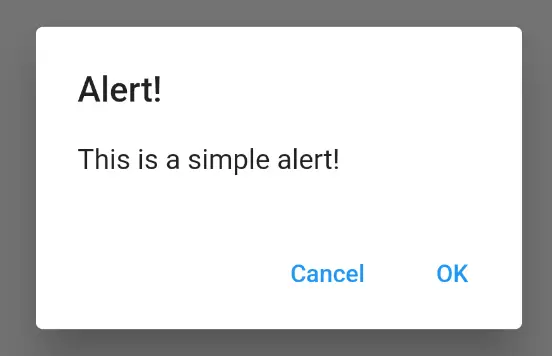
You can change the border radius using the shape property and the RoundedRectangleBorder class. See the AlertDialog code snippet given below.
AlertDialog(
title: const Text('Alert!'),
content: const Text('This is a simple alert!'),
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(30.0),
),
actions: <Widget>[
TextButton(
onPressed: () => Navigator.pop(context, 'Cancel'),
child: const Text('Cancel'),
),
TextButton(
onPressed: () => Navigator.pop(context, 'OK'),
child: const Text('OK'),
),
],
),
Then you will get the following output.
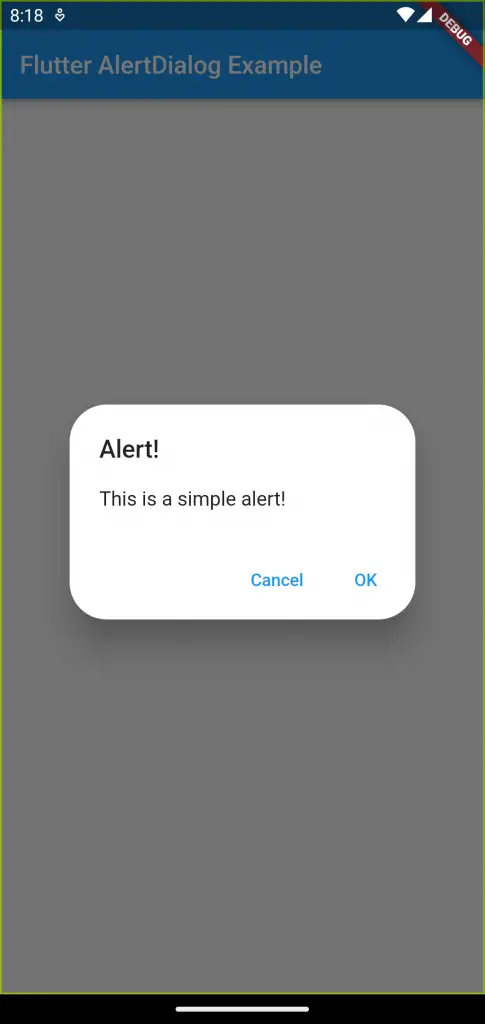
Following is the complete code. The AlertDialog appears when the TextButton is pressed.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter AlertDialog Example'),
),
body: Center(
child: TextButton(
onPressed: () => showDialog<String>(
context: context,
builder: (BuildContext context) => AlertDialog(
title: const Text('Alert!'),
content: const Text('This is a simple alert!'),
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(30.0),
),
actions: <Widget>[
TextButton(
onPressed: () => Navigator.pop(context, 'Cancel'),
child: const Text('Cancel'),
),
TextButton(
onPressed: () => Navigator.pop(context, 'OK'),
child: const Text('OK'),
),
],
),
),
child: const Text('Show Dialog'),
)));
}
}
That’s how you change the AlertDialog border radius in Flutter.