How to change Floating Action Button Elevation in Flutter
The elevation of a component is the distance from the underneath surface. Visually, the elevation results in shadows. In this blog post, let’s check how to change the Floating Action Button elevation in Flutter.
The FloatingActionButton widget has various properties for customization. One among them is elevation. You can change the FAB elevation using it.
See the following code snippet.
FloatingActionButton(
onPressed: () {},
backgroundColor: Colors.amber,
elevation: 20,
child: const Icon(Icons.add),
),
The increased elevation results in highly spread shadows.
See the output given below.
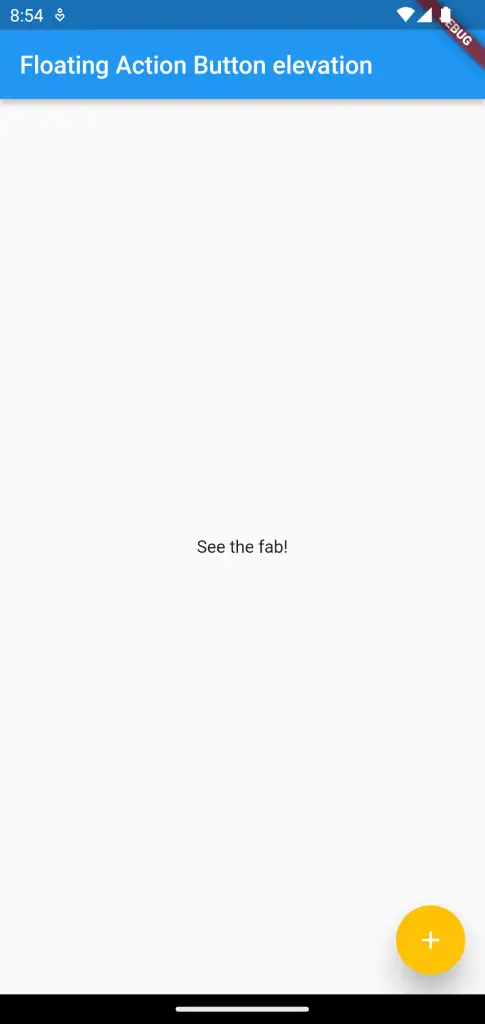
You can even remove shadows using elevation.
Following is the complete code for reference.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Floating Action Button elevation'),
),
body: const Center(child: Text('See the fab!')),
floatingActionButton: FloatingActionButton(
onPressed: () {},
backgroundColor: Colors.amber,
elevation: 20,
child: const Icon(Icons.add),
),
);
}
}
That’s how you change the default elevation of the Floating Action Button in Flutter.