How to change Floating Action Button Size in Flutter
The FloatingActionButton is one of the important button widgets in Flutter. In this blog post, let’s learn how to change the Floating Action Button size in Flutter.
By default, the FloatingActionButton widget has a medium size.
FloatingActionButton(
onPressed: () {},
backgroundColor: Colors.red,
child: const Icon(Icons.add),
),
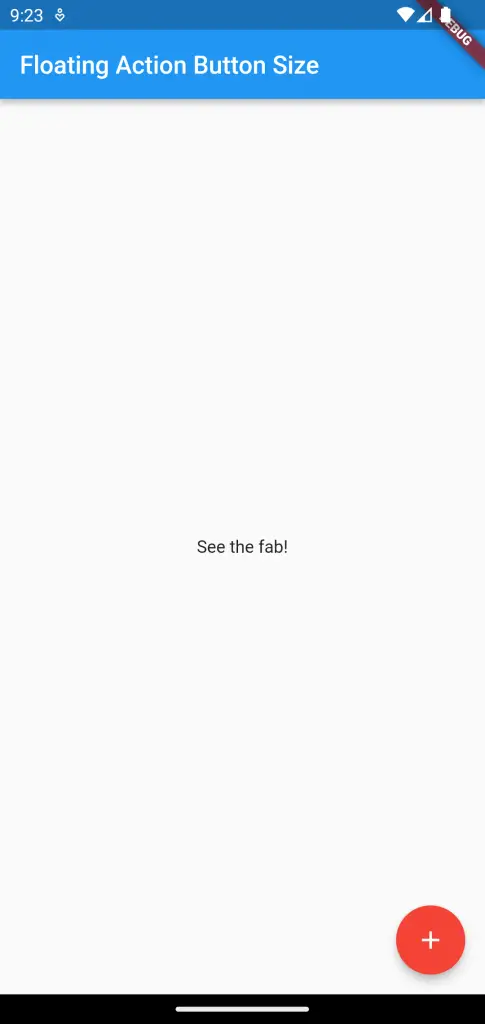
Flutter Floating Action Button Small Size
If you want a FAB with a smaller size than the default size then you can prefer FloatingActionButton.small constructor.
FloatingActionButton.small(
onPressed: () {},
backgroundColor: Colors.red,
child: const Icon(Icons.add),
),
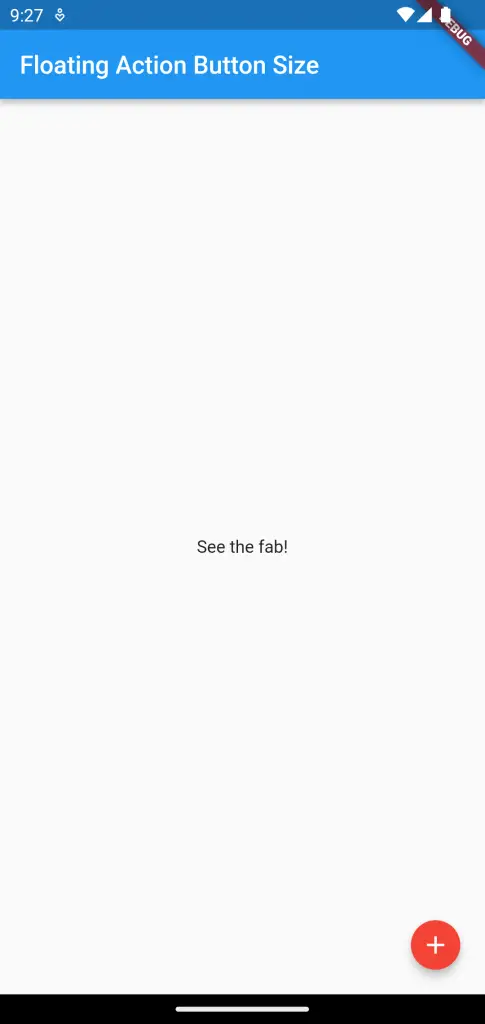
Flutter Floating Action Button Large Size
When the required size of FAB is large then you should go with the FloatingActionButton.large constructor.
FloatingActionButton.large(
onPressed: () {},
backgroundColor: Colors.red,
child: const Icon(Icons.add),
),
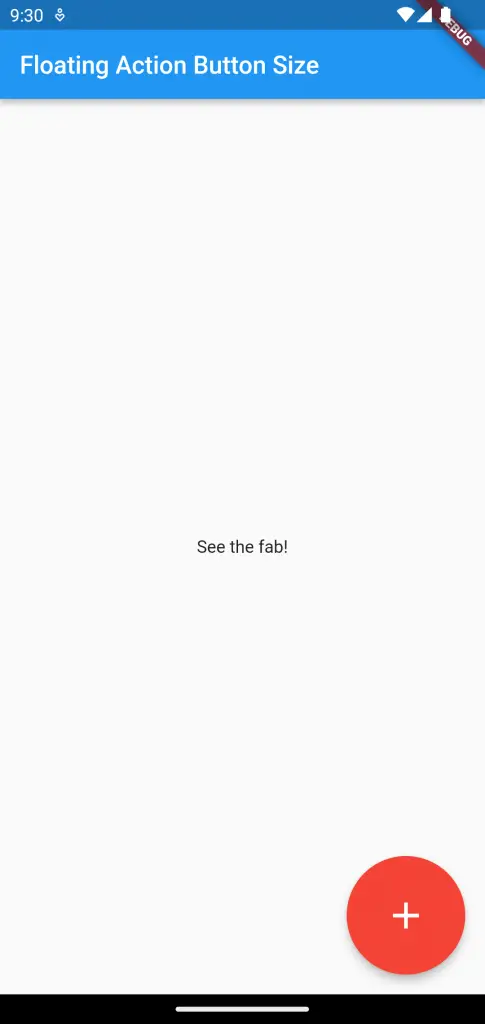
Following is the complete code for reference.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Floating Action Button Size'),
),
body: const Center(child: Text('See the fab!')),
floatingActionButton: FloatingActionButton.large(
onPressed: () {},
backgroundColor: Colors.red,
child: const Icon(Icons.add),
),
);
}
}
Flutter Floating Action Button Custom Size
Sometimes, you may want to set a custom width and height for the FAB. There you should use a SizedBox and make the FAB its child.
See the code snippet given below.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Floating Action Button Size'),
),
body: const Center(child: Text('See the fab!')),
floatingActionButton: SizedBox(
width: 75,
height: 75,
child: FittedBox(
child: FloatingActionButton(
onPressed: () {},
child: const Icon(Icons.add),
),
),
));
}
}
You will get the following output with the given height and width.
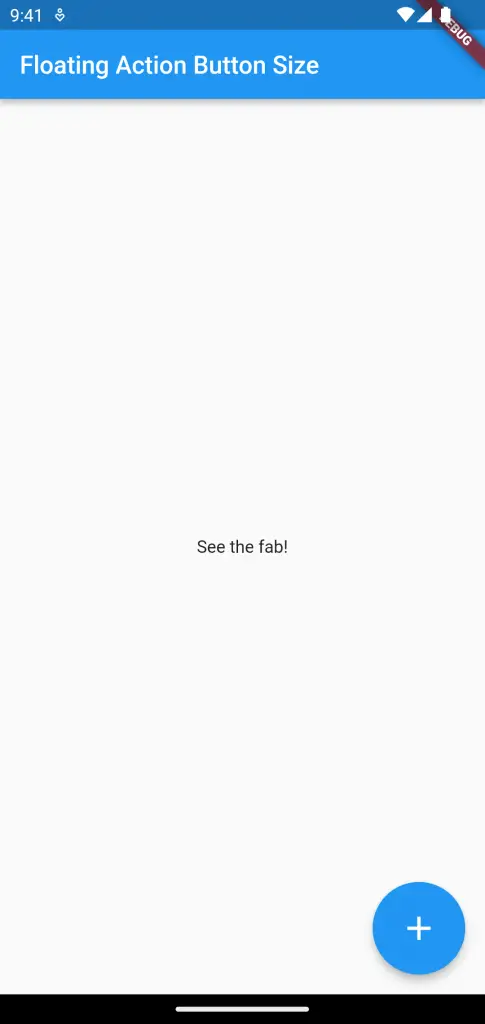
That’s how you change the width and height of the Floating Action Button.