How to change OutlinedButton Background Color in Flutter
OutlinedButton is one of the important widgets in Flutter. As an important UI element, the buttons should have visually appealing colors. In this blog post, let’s learn how to change the OutlinedButton background color in Flutter.
You can customize the OutlinedButton style using OutlinedButton.styleFrom method. You just need to utilize the property backgroundColor. See the code snippet given below.
OutlinedButton(
onPressed: () {},
style: OutlinedButton.styleFrom(backgroundColor: Colors.yellow),
child: const Text('Outlined Button'),
)
You will get the output of OutlinedButton with a yellow color background.
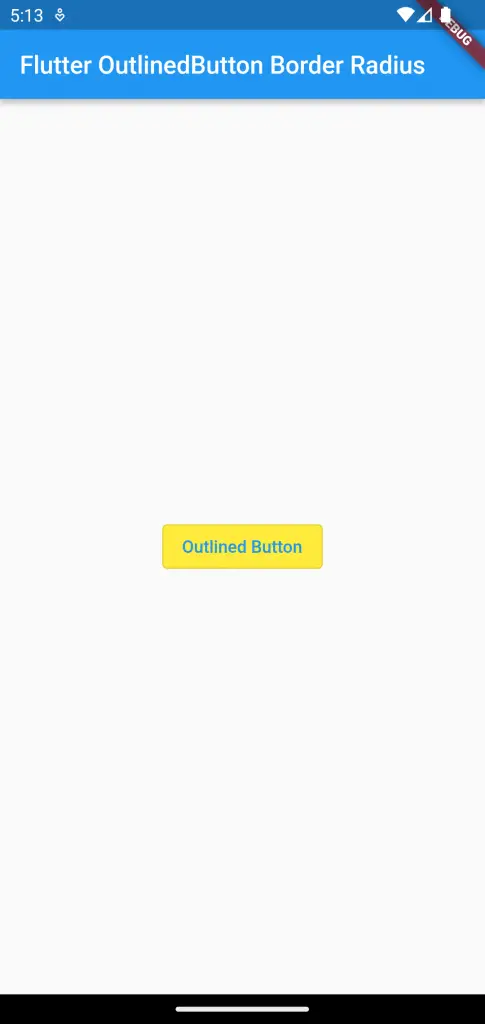
Following is the complete code for reference.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter OutlinedButton Border Radius'),
),
body: Center(
child: OutlinedButton(
onPressed: () {},
style: OutlinedButton.styleFrom(backgroundColor: Colors.yellow),
child: const Text('Outlined Button'),
)));
}
}
If you want to change the border color of the button then see this tutorial.
I hope this Flutter Outlined Button background color tutorial is helpful for you.