How to add Icon Button to AppBar in Flutter
AppBar is an important UI component in a mobile app. In this Flutter tutorial, let’s learn how to add an icon button to AppBar.
The AppBar has actions property which allows displaying a list of widgets in a row after the title. We use actions to add IconButton. We also show a Snackbar when the IconButton is pressed.
AppBar(
title: const Text(
'Flutter AppBar Icon Button',
),
actions: <Widget>[
IconButton(
icon: const Icon(Icons.alarm),
tooltip: 'Show Snackbar',
onPressed: () {
ScaffoldMessenger.of(context).showSnackBar(const SnackBar(
content: Text('This is a Appbar Icon example')));
},
),
]),
Following is the output.
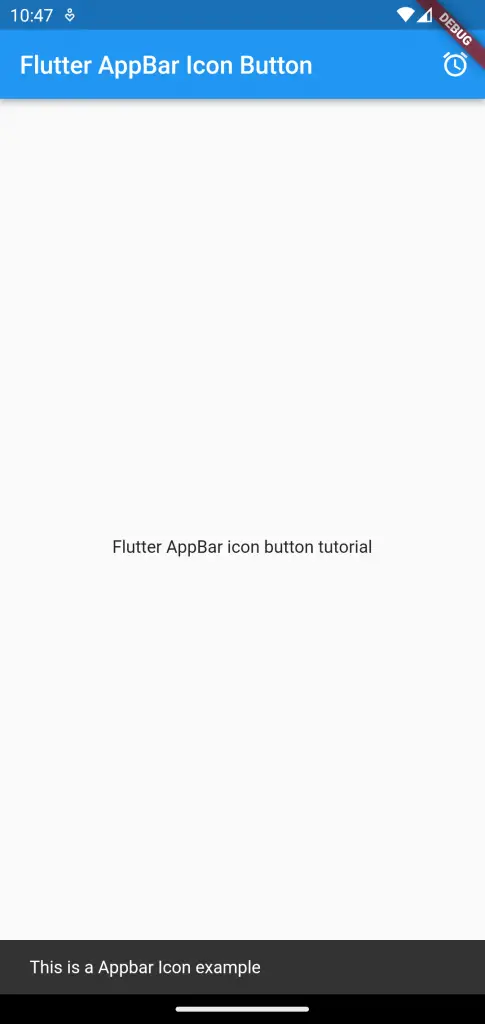
Following is the complete code.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text(
'Flutter AppBar Icon Button',
),
actions: <Widget>[
IconButton(
icon: const Icon(Icons.alarm),
tooltip: 'Show Snackbar',
onPressed: () {
ScaffoldMessenger.of(context).showSnackBar(const SnackBar(
content: Text('This is a Appbar Icon example')));
},
),
]),
body: const Center(child: Text('Flutter AppBar icon button tutorial')));
}
}
That’s how you add IconButton to AppBar in Flutter.