How to add Text Button in Flutter
Flutter has different types of buttons namely- ElevatedButton, OutlinedButton, TextButton, etc. The TextButton in Flutter helps to create button text easily. Let’s jump into this Flutter TextButton tutorial.
The TextButton class is similar to FlatButton which is deprecated. You can pass the Text widget as its child and style the button using styleFrom() method. See the code snippet given below.
TextButton(
style: TextButton.styleFrom(
textStyle: const TextStyle(fontSize: 20),
),
onPressed: () {},
child: const Text('Enabled'),
),
Following is the output.
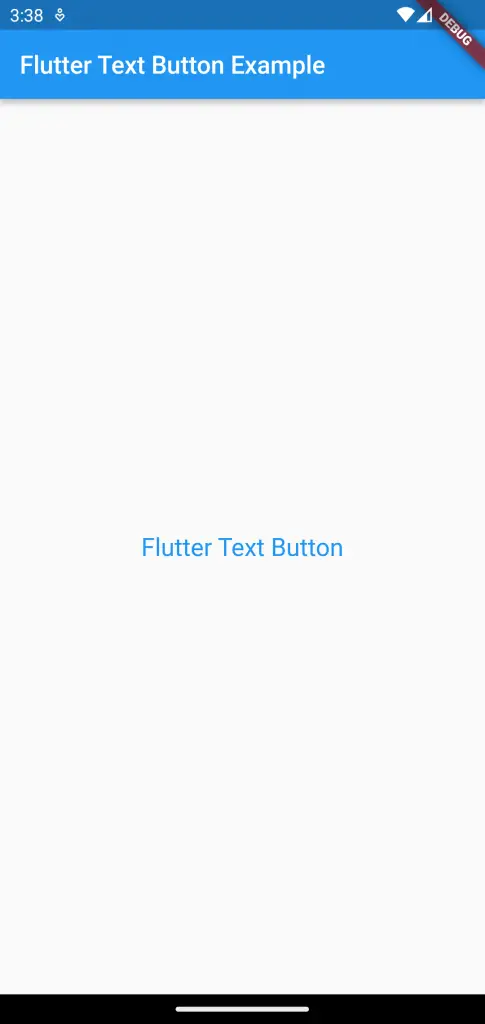
When the TextButton is pressed a ripple effect is shown.
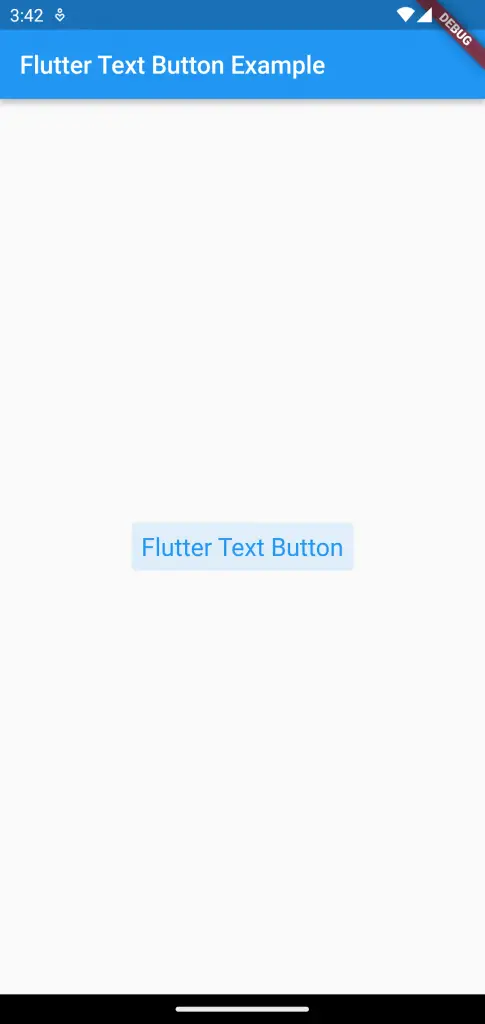
Following is the complete code for Flutter TextButton.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text(
'Flutter Text Button Example',
),
),
body: Center(
child: TextButton(
style: TextButton.styleFrom(
textStyle: const TextStyle(fontSize: 20),
),
onPressed: () {},
child: const Text('Flutter Text Button'),
),
));
}
}
I hope this Flutter Text Button tutorial is helpful for you.