How to add Italic Text in Flutter
Italic text is used to emphasize words or sentences of text content. In this short Flutter tutorial, let’s learn how to create Italic text.
In order to make a Text italic or bold you have to make use of TextStyle. The TextStyle and FontStyle class helps us to make the text Italic. See the following code snippet.
Text(
'This is Flutter Italic Text Tutorial!',
textAlign: TextAlign.center,
style: TextStyle(fontSize: 18, fontStyle: FontStyle.italic),
)
You will get the following output.
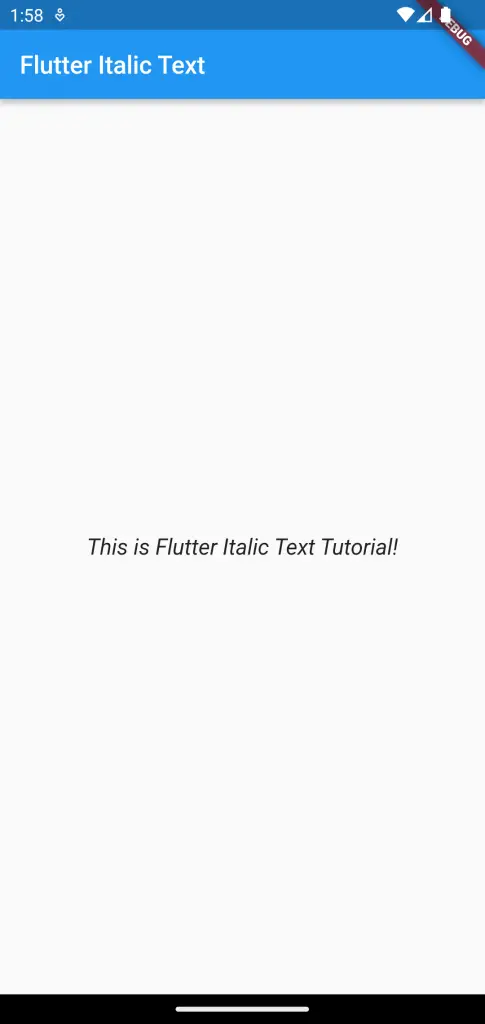
Sometimes, you may want to mix normal and italic words. You should use Text.rich constructor and TextSpan in such cases.
TextSpan(
text: 'This is a ', // default text style
children: <TextSpan>[
TextSpan(
text: ' italic ',
style: TextStyle(fontStyle: FontStyle.italic)),
TextSpan(text: ' text.'),
],
)
Here, only the word italic is made italic.
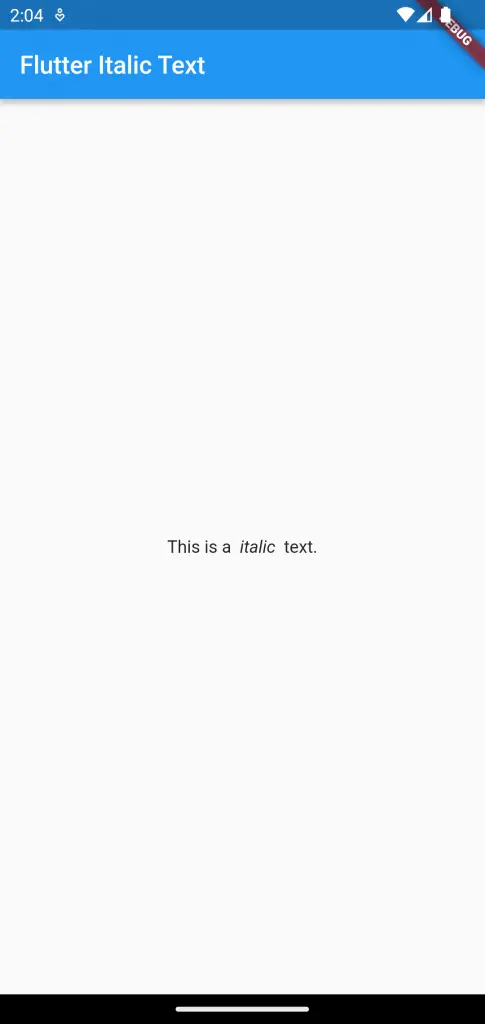
Following is the complete code.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Italic Text'),
),
body: const Center(
child: Text.rich(
TextSpan(
text: 'This is a ', // default text style
children: <TextSpan>[
TextSpan(
text: ' italic ',
style: TextStyle(fontStyle: FontStyle.italic)),
TextSpan(text: ' text.'),
],
),
)),
);
}
}
That’s how you make text italic in Flutter.