How to Load PDF from URL in Flutter
PDFs are integral to many modern applications, allowing users to view and interact with complex documents on their mobile devices. In this blog post, we’ll learn how to load and view a pdf file from a URL in Flutter.
We use the pdfx package to help create a pdf viewer in Flutter. Install the library to your Flutter project using the following command.
flutter pub add pdfx
You also need another dependency internet file for this tutorial. Please install it using the following command.
dart pub add internet_file
You can import both packages as given below.
import 'package:pdfx/pdfx.dart';
import 'package:internet_file/internet_file.dart';
You can open a PDF file from the URL as given below.
PdfDocument.openData(
InternetFile.get('https://www.pdf995.com/samples/pdf.pdf'))
Let’s dive into the complete Flutter code to show PDF files from the internet.
import 'package:flutter/material.dart';
import 'package:pdfx/pdfx.dart';
import 'package:internet_file/internet_file.dart';
void main() => runApp(const MyApp());
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
useMaterial3: true,
),
home: const MyStatefulWidget());
}
}
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({super.key});
@override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
static const int _initialPage = 1;
late PdfController _pdfController;
@override
void initState() {
super.initState();
_pdfController = PdfController(
document: PdfDocument.openData(
InternetFile.get('https://www.pdf995.com/samples/pdf.pdf')),
initialPage: _initialPage,
);
}
@override
void dispose() {
_pdfController.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter PDF Example'),
actions: <Widget>[
IconButton(
icon: const Icon(Icons.navigate_before),
onPressed: () {
_pdfController.previousPage(
curve: Curves.ease,
duration: const Duration(milliseconds: 100),
);
},
),
PdfPageNumber(
controller: _pdfController,
builder: (_, loadingState, page, pagesCount) => Container(
alignment: Alignment.center,
child: Text(
'$page/${pagesCount ?? 0}',
style: const TextStyle(fontSize: 22),
),
),
),
IconButton(
icon: const Icon(Icons.navigate_next),
onPressed: () {
_pdfController.nextPage(
curve: Curves.ease,
duration: const Duration(milliseconds: 100),
);
},
),
],
),
body: PdfView(
builders: PdfViewBuilders<DefaultBuilderOptions>(
options: const DefaultBuilderOptions(),
documentLoaderBuilder: (_) =>
const Center(child: CircularProgressIndicator()),
pageLoaderBuilder: (_) =>
const Center(child: CircularProgressIndicator()),
),
controller: _pdfController,
),
);
}
}
The initState method is called when the widget is first created and is used to initialize the _pdfController field. This field is an instance of the PdfController class, which is used to control the PDF document.
The PdfController is created with a PdfDocument object, which is obtained by calling InternetFile.get with a URL string.
The AppBar has a title and a list of icons, which can be used to navigate between pages in the PDF document. The body of the Scaffold is a PdfView widget, which displays the PDF document.
The PdfView has builders and a controller property. The builders property defines the widgets that are used to display the PDF document when it is being loaded, or when there is an error. The controller property is the PdfController instance that was created in the initState method.
Finally, the dispose method is called when the widget is no longer in use and is used to clean up any resources that were acquired during the lifetime of the widget.
Following is the output.
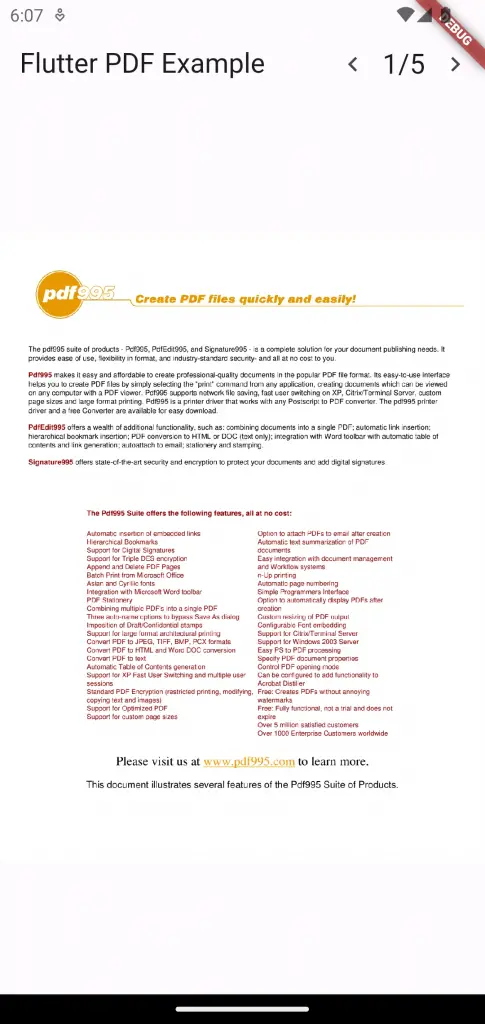
I hope this Flutter load PDF from the URL is helpful for you.