How to change ElevatedButton Opacity in Flutter
As a mobile app developer, you may tweak the opacity of UI components for multiple purposes. In this Flutter tutorial, let’s learn how to change the default opacity of ElevatedButton.
You can customize the background color of ElevatedButton by using the backgroundColor property of the ButtonStyle class. There we can change the opacity of the button using the withOpacity method.
See the code snippet given below.
ElevatedButton(
onPressed: () {},
style: ElevatedButton.styleFrom(
elevation: 0,
backgroundColor: Colors.red.withOpacity(0.1),
foregroundColor: Colors.black,
side: const BorderSide(
width: 1.0,
color: Colors.green,
)),
child: const Text(' Elevated Button'))
Make sure the elevation of ElevateButton is zero. The value of opacity should between 0.0 to 1.0. If you want you can also apply opacity to the foreground color too.
The output is given below.
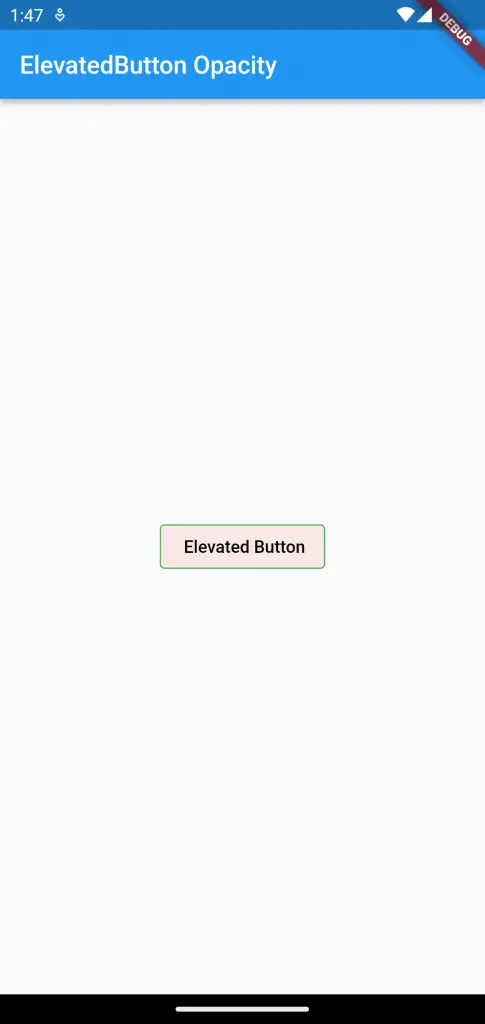
Following is the complete code for reference.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('ElevatedButton Opacity'),
),
body: Center(
child: ElevatedButton(
onPressed: () {},
style: ElevatedButton.styleFrom(
elevation: 0,
backgroundColor: Colors.red.withOpacity(0.1),
foregroundColor: Colors.black,
side: const BorderSide(
width: 1.0,
color: Colors.green,
)),
child: const Text(' Elevated Button'))));
}
}
I hope this Flutter ElevatedButton opacity tutorial is helpful for you.