How to change Checkbox Border Width in Flutter
The CheckBox is an important widget in Flutter. Hence, one should style the CheckBox properly. In this Flutter tutorial, let’s learn how to change the CheckBox border width.
The CheckBox widget comes with a side property to customize border color, border width, etc. The MaterialStateBorder class helps to choose border width for different Material states.
See the code snippet given below.
Checkbox(
side: MaterialStateBorderSide.resolveWith(
(Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return const BorderSide(width: 2, color: Colors.red);
}
return const BorderSide(width: 1, color: Colors.red);
},
),
value: checkBoxValue,
onChanged: (bool? newValue) {
setState(() {
checkBoxValue = newValue!;
});
})
The border width is 2 when the checkbox is selected where as it is 1 for all other states.
The following is the output.
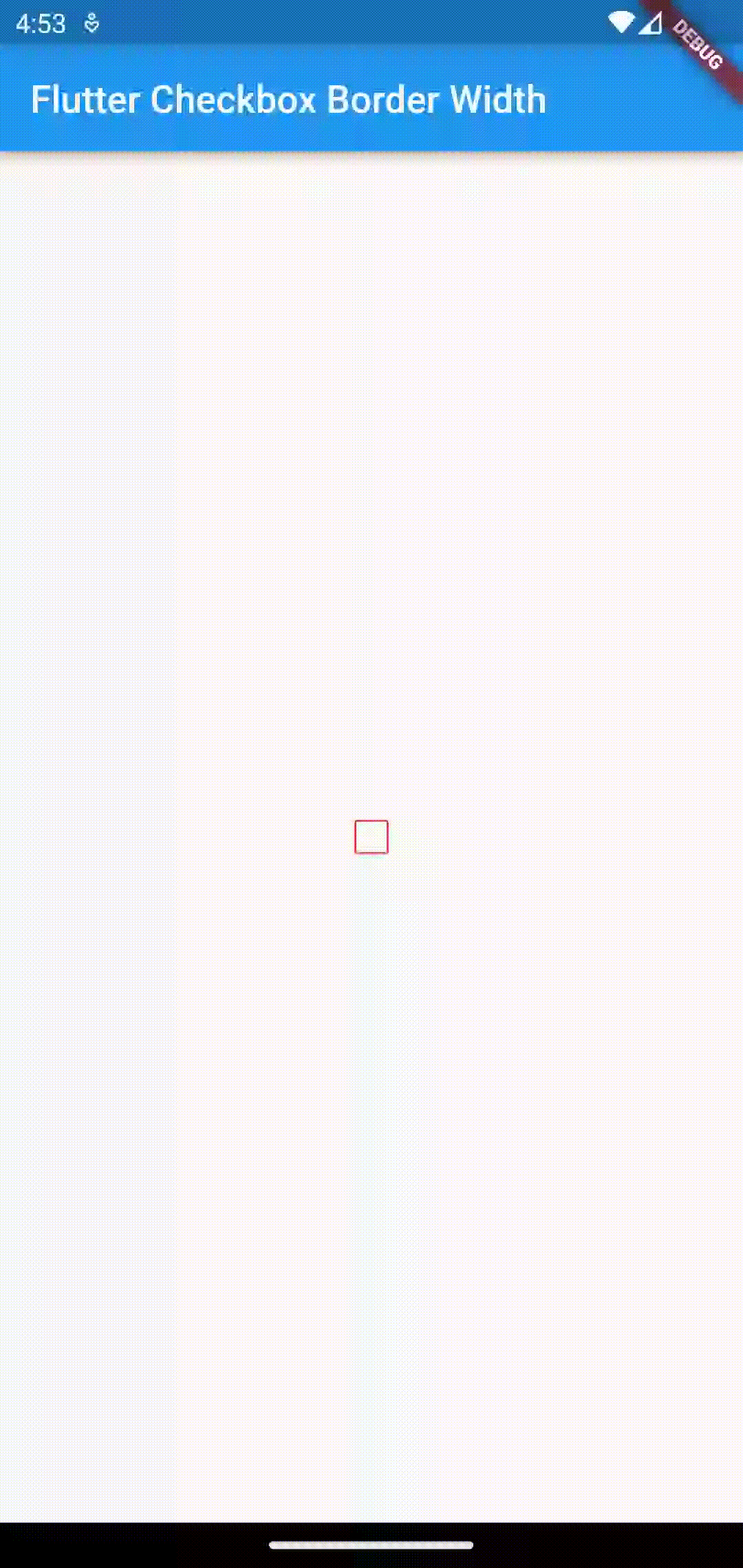
The complete code is given below.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Checkbox Border Width'),
),
body: const MyStatefulWidget());
}
}
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({super.key});
@override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
bool checkBoxValue = false;
@override
Widget build(BuildContext context) {
return Center(
child: Checkbox(
side: MaterialStateBorderSide.resolveWith(
(Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return const BorderSide(width: 2, color: Colors.red);
}
return const BorderSide(width: 1, color: Colors.red);
},
),
value: checkBoxValue,
onChanged: (bool? newValue) {
setState(() {
checkBoxValue = newValue!;
});
}));
}
}
That’s how you change default border width of CheckBox in Flutter.