How to change Checkbox Border Color in Flutter
The checkboxes are important UI components in a mobile app. In Flutter, we use the CheckBox widget to create checkboxes. In this blog post, let’s learn how to change the CheckBox border color in Flutter.
The CheckBox widget has a side property to define the border. It accepts MaterialStateBorderSide class and hence you can give different border colors for different Material states.
See the code snippet given below.
Checkbox(
side: MaterialStateBorderSide.resolveWith(
(Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return const BorderSide(color: Colors.red);
}
return const BorderSide(color: Colors.green);
},
),
value: checkBoxValue,
onChanged: (bool? newValue) {
setState(() {
checkBoxValue = newValue!;
});
})
Here, a red color border is given when the checkbox is selected. And for all other states, we have given green border color.
Following is the output.
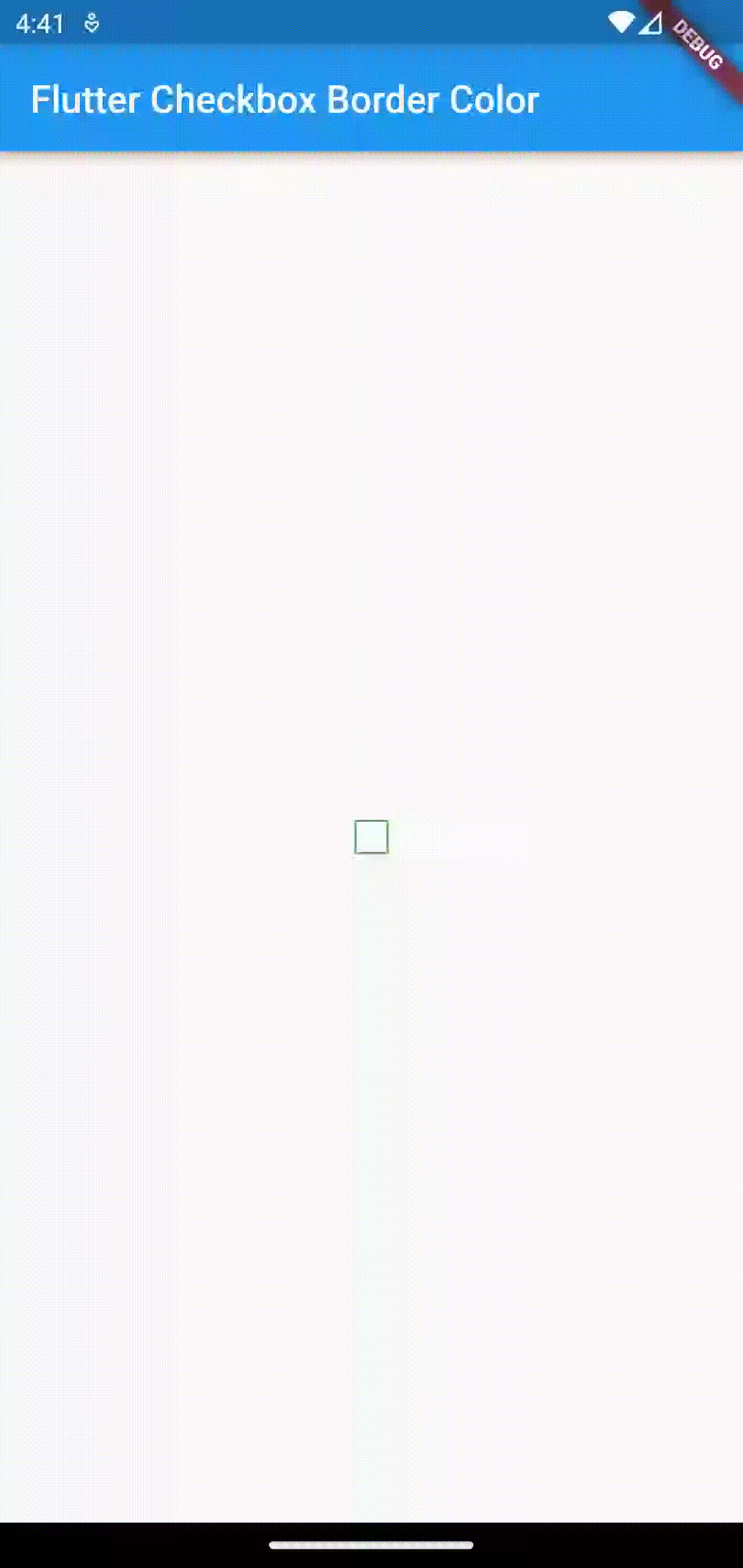
If you want to learn more about checkbox color, see this tutorial on Flutter CheckBox color.
Following is the complete code.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Checkbox Border Color'),
),
body: const MyStatefulWidget());
}
}
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({super.key});
@override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
bool checkBoxValue = false;
@override
Widget build(BuildContext context) {
return Center(
child: Checkbox(
side: MaterialStateBorderSide.resolveWith(
(Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return const BorderSide(color: Colors.red);
}
return const BorderSide(color: Colors.green);
},
),
value: checkBoxValue,
onChanged: (bool? newValue) {
setState(() {
checkBoxValue = newValue!;
});
}));
}
}
That’s how you change CheckBox border color in Flutter.