How to change Checkbox Color in Flutter
The CheckBox widget in Flutter helps to display a check box that can be toggled by the user. In this blog post, let’s learn how to set CheckBox color in Flutter.
The CheckBox widget has some useful properties for customization. The activeColor property helps to change the color of the checkbox when it’s active. The checkColor property helps to change the color of the tick mark.
See the following code snippet.
Checkbox(
value: checkBoxValue,
activeColor: Colors.red,
checkColor: Colors.white,
onChanged: (bool? newValue) {
setState(() {
checkBoxValue = newValue!;
});
})
Then you will get the following output.
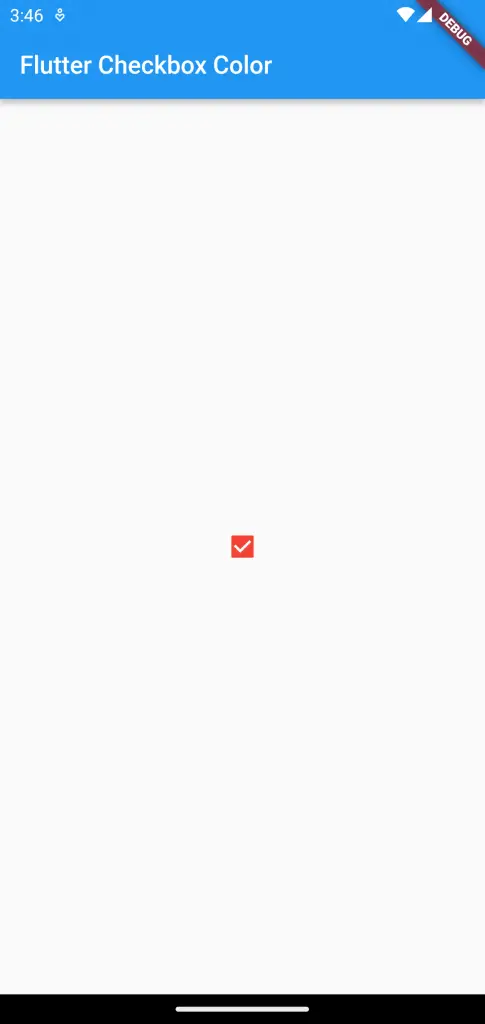
Most of the time, you may need more control over the checkbox color. Then you should choose the fillColor property. You can customize the colors according to the Material state of the CheckBox.
See the code snippet given below.
Color getColor(Set<MaterialState> states) {
const Set<MaterialState> interactiveStates = <MaterialState>{
MaterialState.selected,
MaterialState.focused,
MaterialState.pressed,
};
if (states.any(interactiveStates.contains)) {
return Colors.orange;
}
return Colors.pink;
}
return Center(
child: Checkbox(
checkColor: Colors.black,
fillColor: MaterialStateProperty.resolveWith(getColor),
value: checkBoxValue,
onChanged: (bool? newValue) {
setState(() {
checkBoxValue = newValue!;
});
}));
Then you get the following output.
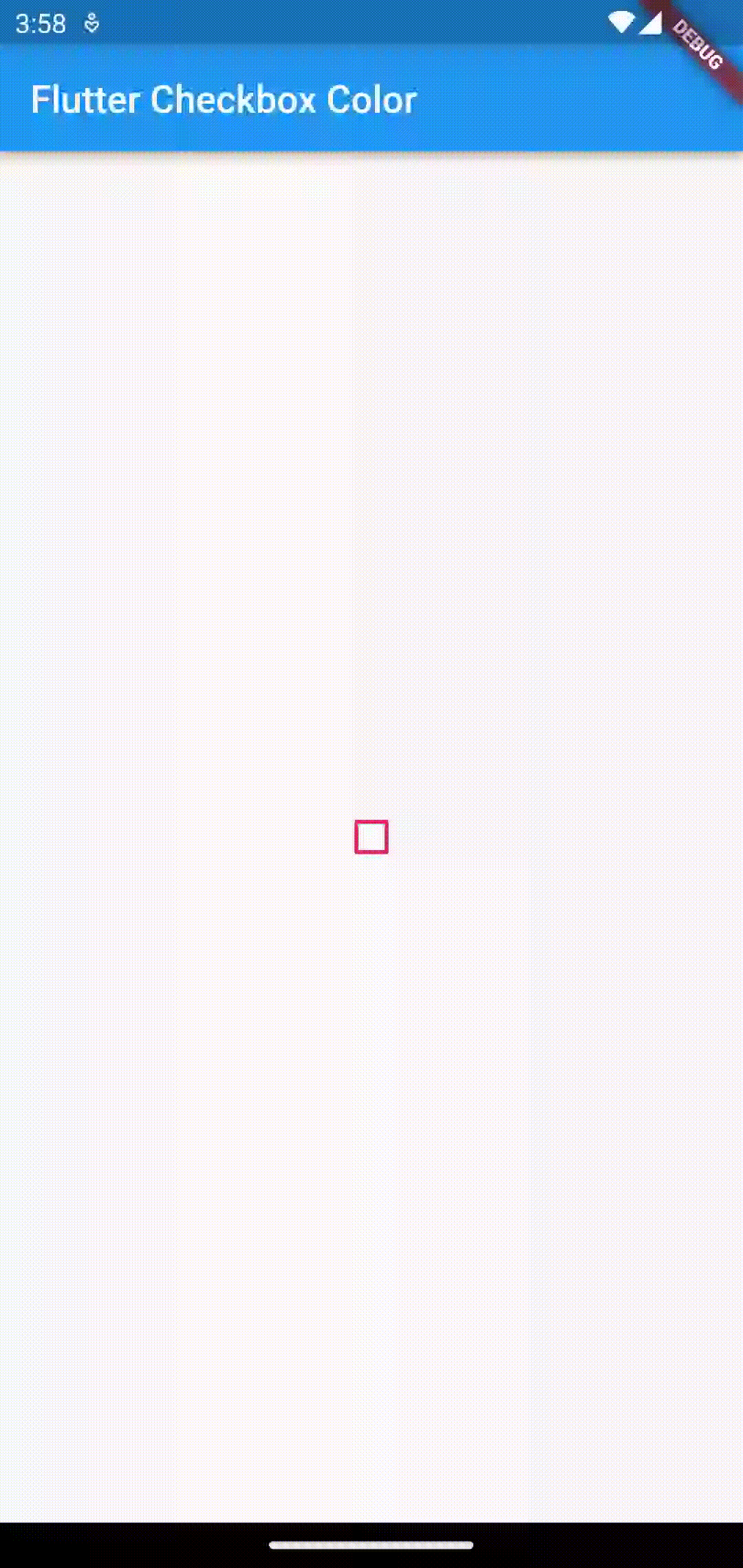
Following is the complete code.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Checkbox Color'),
),
body: const MyStatefulWidget());
}
}
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({super.key});
@override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
bool checkBoxValue = false;
@override
Widget build(BuildContext context) {
Color getColor(Set<MaterialState> states) {
const Set<MaterialState> interactiveStates = <MaterialState>{
MaterialState.selected,
MaterialState.focused,
MaterialState.pressed,
};
if (states.any(interactiveStates.contains)) {
return Colors.orange;
}
return Colors.pink;
}
return Center(
child: Checkbox(
checkColor: Colors.black,
fillColor: MaterialStateProperty.resolveWith(getColor),
value: checkBoxValue,
onChanged: (bool? newValue) {
setState(() {
checkBoxValue = newValue!;
});
}));
}
}
That’s how you change CheckBox color in Flutter.