How to change Checkbox Size in Flutter
The CheckBox is an important widget in Flutter. It helps users to give input just by toggling. In this blog post, let’s learn how to set CheckBox size in Flutter.
Even though the CheckBox widget has many useful properties, it doesn’t have any property to change the size. Hence we should depend on other widgets to change the default checkbox size.
Here, we use the Transform widget and scale the child to increase the size.
See the code snippet given below.
Transform.scale(
scale: 1.5,
child: Checkbox(
value: checkBoxValue,
onChanged: (bool? newValue) {
setState(() {
checkBoxValue = newValue!;
});
}))
The value of the scale is 1.5, which means it’s 1.5x the default size.
The following is the output.
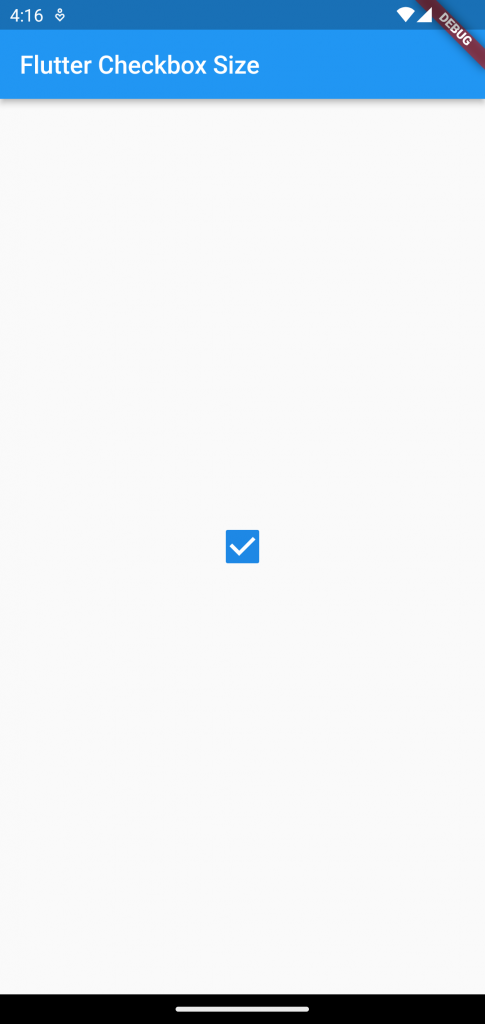
Following is the complete code.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Checkbox Size'),
),
body: const MyStatefulWidget());
}
}
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({super.key});
@override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
bool checkBoxValue = false;
@override
Widget build(BuildContext context) {
return Center(
child: Transform.scale(
scale: 1.5,
child: Checkbox(
value: checkBoxValue,
onChanged: (bool? newValue) {
setState(() {
checkBoxValue = newValue!;
});
})));
}
}
That’s how you change CheckBox size in Flutter.