How to Place a Button at the Top of the Screen in Flutter
Alignment is an important aspect while designing your mobile app user interface. Sometimes, you may want to align your widget at the top of the screen in Flutter. In this blog post, I am going to show you how to add a button and place it at the top of the screen in Flutter.
I have already posted about how to align a button at the bottom of the screen in Flutter. For aligning a widget you have to make it as a child of Align widget. Using the alignment property of Align class as well as the Alignment class you can align the widget at the top of the screen.
In the following Flutter example, I have a RaisedButton widget which is aligned top-center to the screen.
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Welcome to Flutter',
home: Scaffold(
appBar: AppBar(
title: Text('Flutter button at the top example'),
),
body: Center(child: Body()),
),
);
}
}
/// This is the stateless widget that the main application instantiates.
class Body extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Align(
alignment: Alignment.topCenter,
child: RaisedButton(
onPressed: () {},
child: const Text('Top Button!', style: TextStyle(fontSize: 20)),
color: Colors.blue,
textColor: Colors.white,
elevation: 5,
),
);
}
}
The following is the output of this Flutter example.
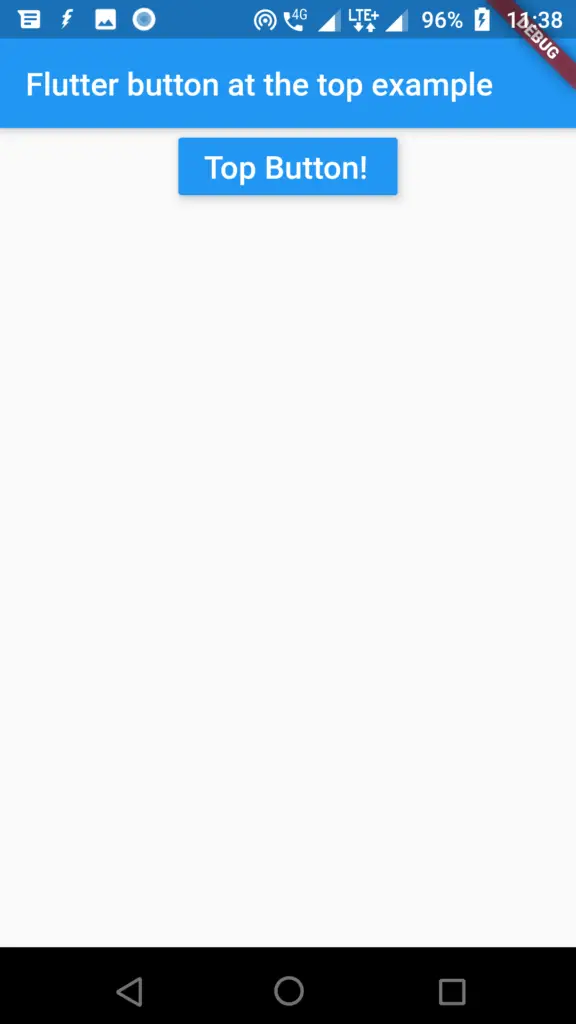