How to Show SnackBar in Flutter
In Material Design, a SnackBar shows the user briefly some kind of useful information. Having SnackBar in your mobile application will really help users to get information about their actions inside the app. In this blog post, let’s see how to add and display SnackBar in Flutter.
The SnackBar class is needed to create a snack bar widget in Flutter. You can create a simple snack bar as given in the snippet below:
final snackBar = SnackBar(
content: const Text('Hello! I am a SnackBar!'),
duration: const Duration(seconds: 5),
action: SnackBarAction(
label: 'Undo',
onPressed: () {
// Some code to undo the change.
},
),
);
As you see, the properties of the SnackBar class are really understandable. You can define the content, duration, and action of the snack bar easily.
After defining, you can show the SnackBar using the snippet given below:
ScaffoldMessenger.of(context).showSnackBar(snackBar);
Following is the complete Flutter tutorial to show SnackBar. While Pressing the ElevatedButton the snack bar appears for the given duration.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Snackbar example'),
),
body: Center(
child: ElevatedButton(
onPressed: () {
final snackBar = SnackBar(
content: const Text('Hello! I am a SnackBar!'),
duration: const Duration(seconds: 5),
action: SnackBarAction(
label: 'Undo',
onPressed: () {
// Some code to undo the change.
},
),
);
ScaffoldMessenger.of(context).showSnackBar(snackBar);
},
child: const Text('Click Me!'),
),
));
}
}
Following is the output of the Flutter SnackBar example.
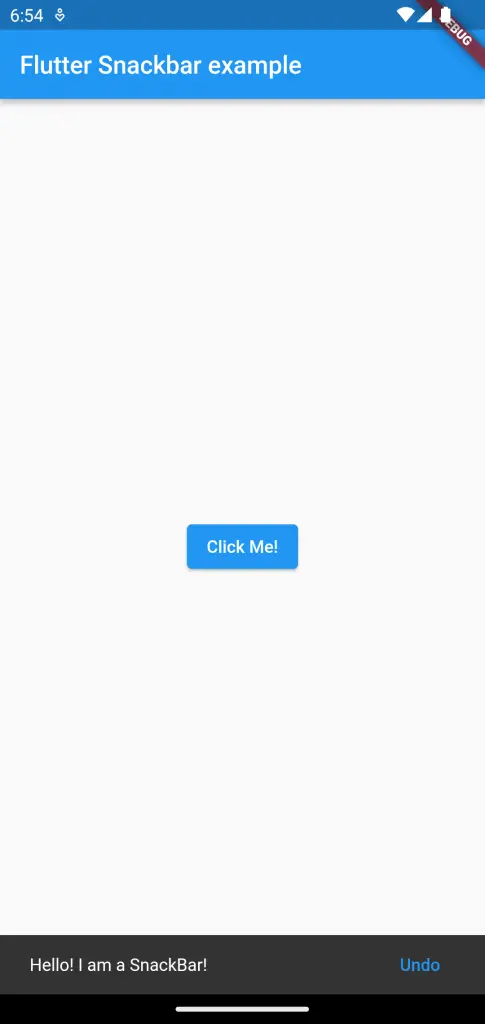
That’s how you show a simple snackbar in Flutter.