How to add Background Color to TextButton in Flutter
TextButton is one of the most important button widgets of Flutter. There are many ways to customize a TextButton. In this Flutter tutorial, let’s learn how to set the TextButton background color.
TextButton.styleFrom() method can be used to style the TextButton. It has many properties and backgroundColor is one among them to change the text button background color. Following is the code snippet.
TextButton(
style: TextButton.styleFrom(
padding: const EdgeInsets.all(10),
foregroundColor: Colors.yellow,
backgroundColor: Colors.green,
textStyle: const TextStyle(fontSize: 20)),
onPressed: () {},
child: const Text('TextButton Background'),
),
Here, the yellow color is the text color and the green color is the background. See the output given below.
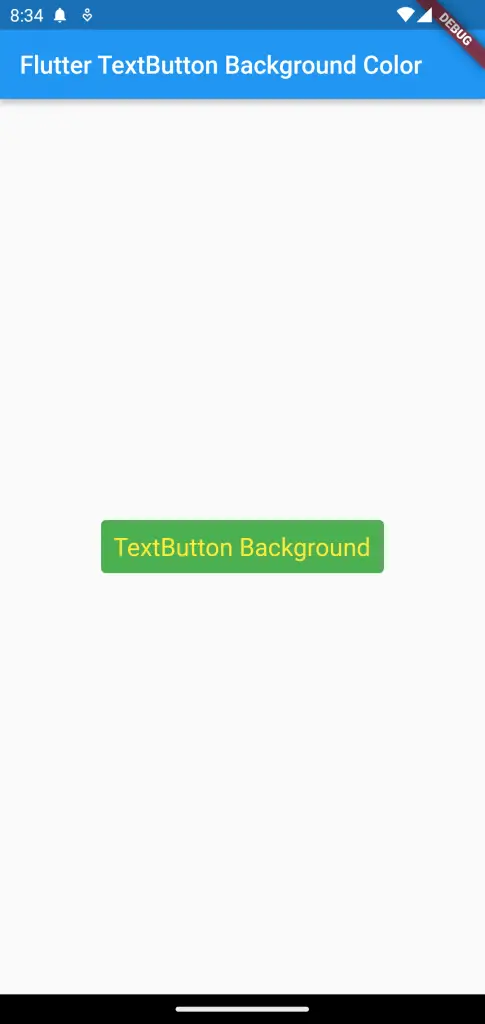
Following is the complete code.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text(
'Flutter TextButton Background Color',
),
),
body: Center(
child: TextButton(
style: TextButton.styleFrom(
padding: const EdgeInsets.all(10),
foregroundColor: Colors.yellow,
backgroundColor: Colors.green,
textStyle: const TextStyle(fontSize: 20)),
onPressed: () {},
child: const Text('TextButton Background'),
),
));
}
}
That’s how you change the TextButton background color in Flutter.