How to set Height and Width for TextButton in Flutter
TextButton is one of the popular Flutter widgets available to create buttons. By default, the size of TextButton depends on the text size and other factors such as padding. Let’s learn how to set fixed height and width for TextButton in this Flutter tutorial.
In order to set a fixed size for TextButton, it provides a property named fixedSize. You can set the predefined width and height of TextButton by making use of fixedSize property and Size class.
See the code snippet given below.
TextButton(
style: TextButton.styleFrom(
fixedSize: const Size(300, 100),
foregroundColor: Colors.red,
backgroundColor: Colors.yellow,
textStyle: const TextStyle(fontSize: 20)),
onPressed: () {},
child: const Text('TextButton width & height'),
),
We have given a width of 300 and a height of 100. The output is given below.
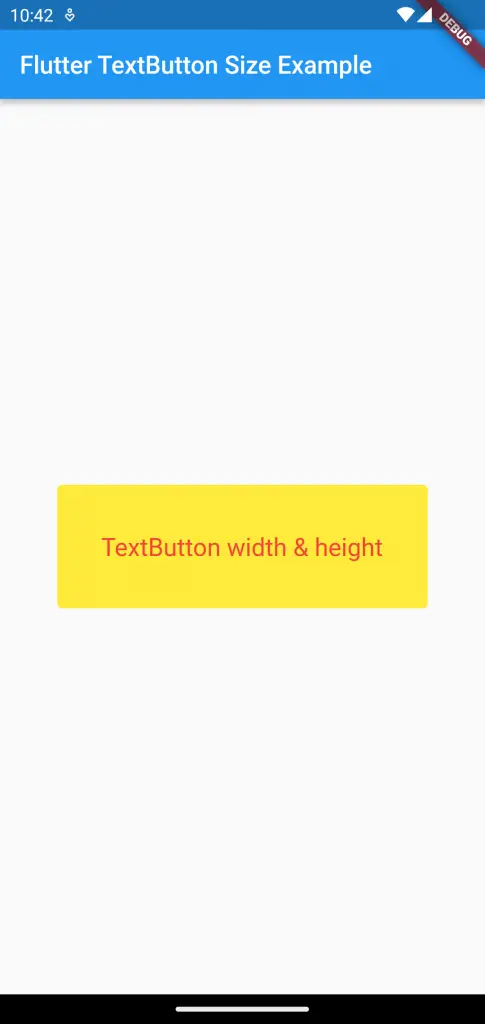
Following is the complete code for the Flutter TextButton size example.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text(
'Flutter TextButton Size Example',
),
),
body: Center(
child: TextButton(
style: TextButton.styleFrom(
fixedSize: const Size(300, 100),
foregroundColor: Colors.red,
backgroundColor: Colors.yellow,
textStyle: const TextStyle(fontSize: 20)),
onPressed: () {},
child: const Text('TextButton width & height'),
),
));
}
}
That’s how you create a TextButton with fixed width and height in Flutter.