How to Justify Text in Flutter
Proper alignment of UI elements is a must for a good-looking mobile app. We always want to align text too so that it appears neatly. In this Flutter tutorial, let’s learn how to justify text in Flutter.
Firstly, let’s see how text appears without justification.
const Text(
'This is a simple flutter tutorial to show how to justify text'
'See the result in the given screen. Thats it. Thank you for reading! ',
style: TextStyle(
fontSize: 18,
)),
The output will be as given below.
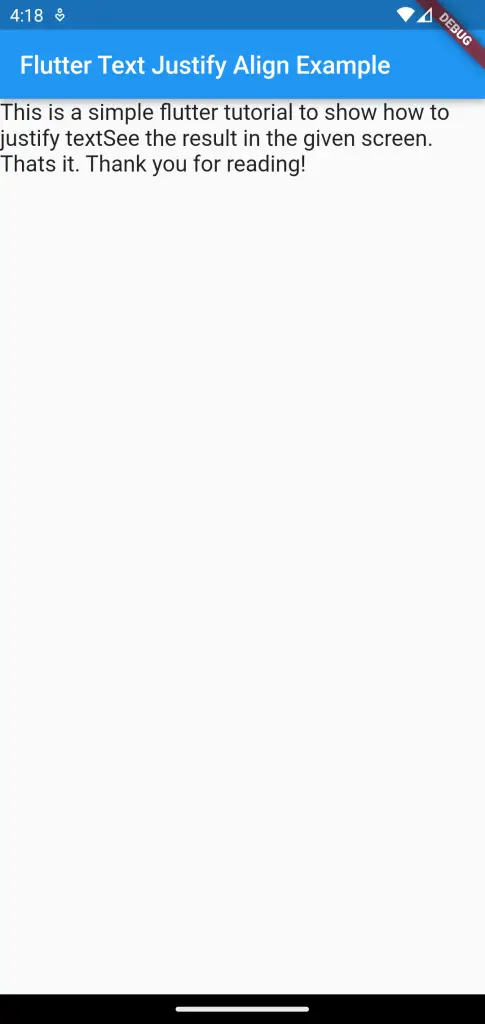
Justifying text will add spaces between words to fit into the available width. You can justify text in Flutter using textAlign property of the Text widget. See the code snippet given below.
const Text(
'This is a simple flutter tutorial to show how to justify text'
'See the result in the given screen. Thats it. Thank you for reading! ',
textAlign: TextAlign.justify,
style: TextStyle(
fontSize: 18,
)),
Now, see the output.
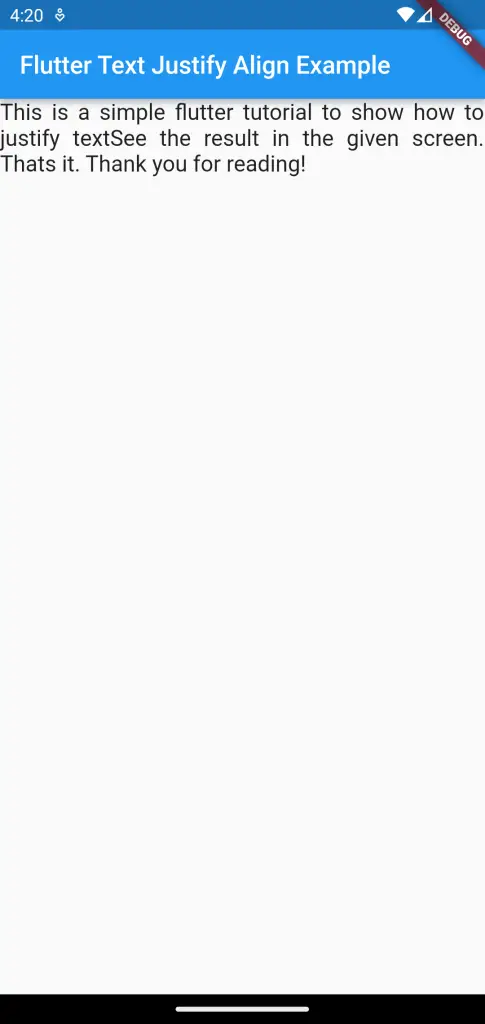
Following is the complete code for reference.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Text Justify Align Example'),
),
body: const Text(
'This is a simple flutter tutorial to show how to justify text'
'See the result in the given screen. Thats it. Thank you for reading! ',
textAlign: TextAlign.justify,
style: TextStyle(
fontSize: 18,
)),
);
}
}
I hope this Flutter text justify tutorial is helpful for you.