How to add Text Line Break in Flutter
Line breaks are helpful to break text into multiple lines so that users can read the text properly. Let’s check how to add a line break for the Text widget in Flutter.
In order to create line breaks, you just need to add \n to your text content. You have to insert \n and the line breaks from there. See the following code snippet.
Text('Break this line \n into two.',
style: TextStyle(fontSize: 18, color: Colors.red)),
),
Now, see the output given below.
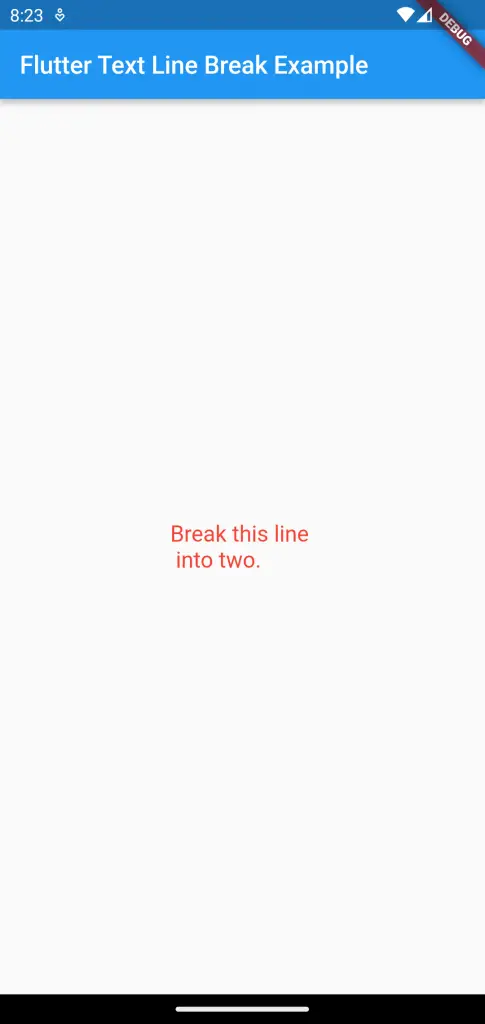
As you see, the line breaks from where \n is added. The complete code of this Flutter example is given below.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Text Line Break Example'),
),
body: const Center(
child: Text('Break this line \n into two.',
style: TextStyle(fontSize: 18, color: Colors.red)),
),
);
}
}
That’s how you add line breaks in Flutter.