How to position CheckboxListTile Checkbox to Left in Flutter
The CheckboxListTile widget in Flutter helps to display checkboxes on a tile with text, icons, etc. In this blog post, let’s learn how to bring the CheckboxListTile checkbox to the left in Flutter.
By default, the checkbox of the CheckboxListTile widget appears at the right. You can change the position of the checkbox using the controlAffinity property.
See the code snippet given below.
CheckboxListTile(
title: const Text('Checkbox with icon & text'),
controlAffinity: ListTileControlAffinity.leading,
secondary: const Icon(
Icons.face,
color: Colors.blue,
),
value: checkBoxValue,
onChanged: (bool? newValue) {
setState(() {
checkBoxValue = newValue!;
});
},
)
The ListTileControlAffinity.leading makes the checkbox move to the left position.
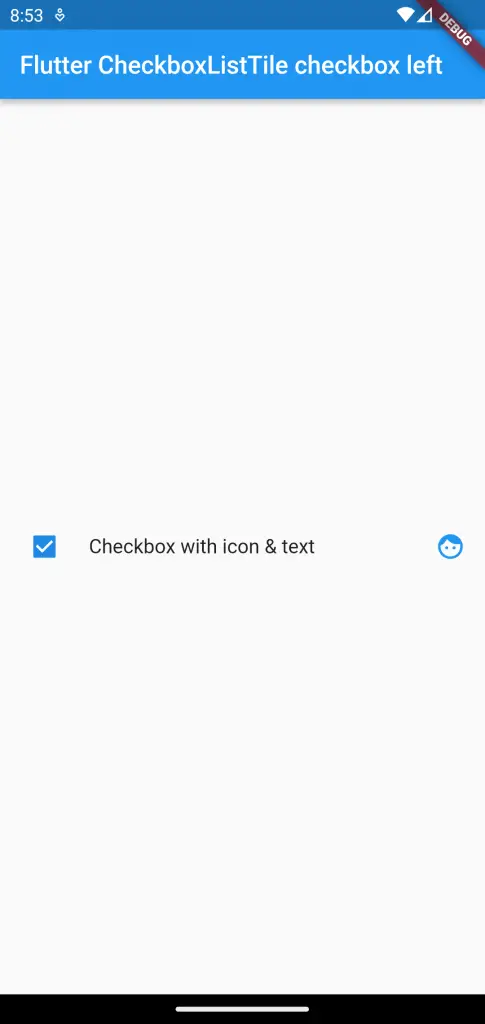
Following is the complete code.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter CheckboxListTile checkbox left'),
),
body: const MyStatefulWidget());
}
}
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({super.key});
@override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
bool checkBoxValue = false;
@override
Widget build(BuildContext context) {
return Center(
child: CheckboxListTile(
title: const Text('Checkbox with icon & text'),
controlAffinity: ListTileControlAffinity.leading,
secondary: const Icon(
Icons.face,
color: Colors.blue,
),
value: checkBoxValue,
onChanged: (bool? newValue) {
setState(() {
checkBoxValue = newValue!;
});
},
),
);
}
}
That’s how you change the position of CheckboxListTile checkbox to left in Flutter.