How to set CheckboxListTile Background Color in Flutter
The CheckboxListTile widget in Flutter is a mix of the Checkbox and the ListTile widgets. In this blog post, let’s learn how to change CheckboxListTile background color in Flutter.
The CheckboxList widget has some useful properties for customization. We use the tileColor property to change the background color of the tile.
See the code snippet given below.
CheckboxListTile(
title: const Text('Checkbox with text'),
controlAffinity: ListTileControlAffinity.leading,
tileColor: Colors.yellow,
value: checkBoxValue,
onChanged: (bool? newValue) {
setState(() {
checkBoxValue = newValue!;
});
},
)
You will get the output with the yellow background color.
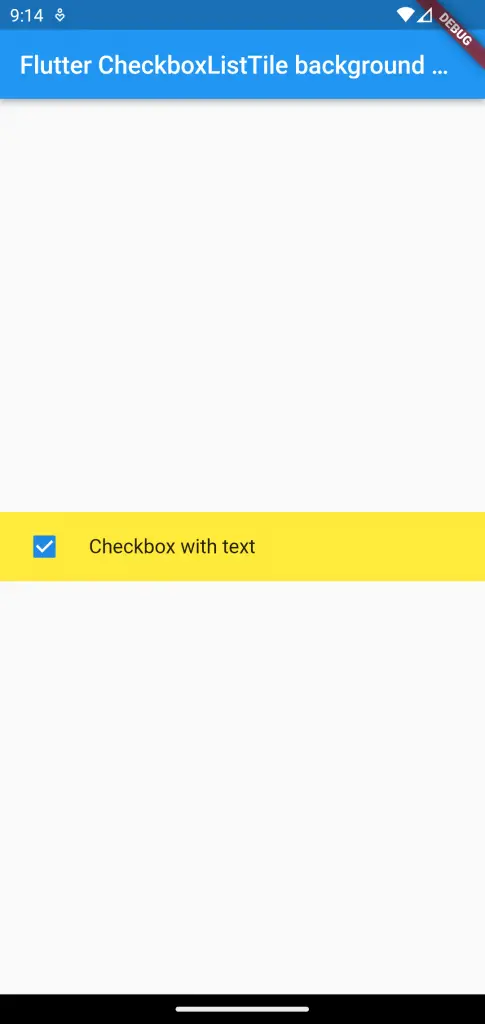
Following is the complete code.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter CheckboxListTile background color'),
),
body: const MyStatefulWidget());
}
}
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({super.key});
@override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
bool checkBoxValue = false;
@override
Widget build(BuildContext context) {
return Center(
child: CheckboxListTile(
title: const Text('Checkbox with text'),
controlAffinity: ListTileControlAffinity.leading,
tileColor: Colors.yellow,
value: checkBoxValue,
onChanged: (bool? newValue) {
setState(() {
checkBoxValue = newValue!;
});
},
),
);
}
}
That’s how you set CheckboxListTile background color in Flutter.