How to create Round Checkbox in Flutter
The CheckBox widget is one of the commonly used widgets in Flutter. It helps users input data by toggling the box. In this tutorial, let’s check how to create a round CheckBox in Flutter.
The CheckBox has shape property. The shape property helps to tweak the shape of the checkbox. We can make the CheckBox circular using the CircleBorder class.
See the following code snippet.
Checkbox(
shape: const CircleBorder(),
value: checkBoxValue,
onChanged: (bool? newValue) {
setState(() {
checkBoxValue = newValue!;
});
},
),
You will get an output of a circle CheckBox.
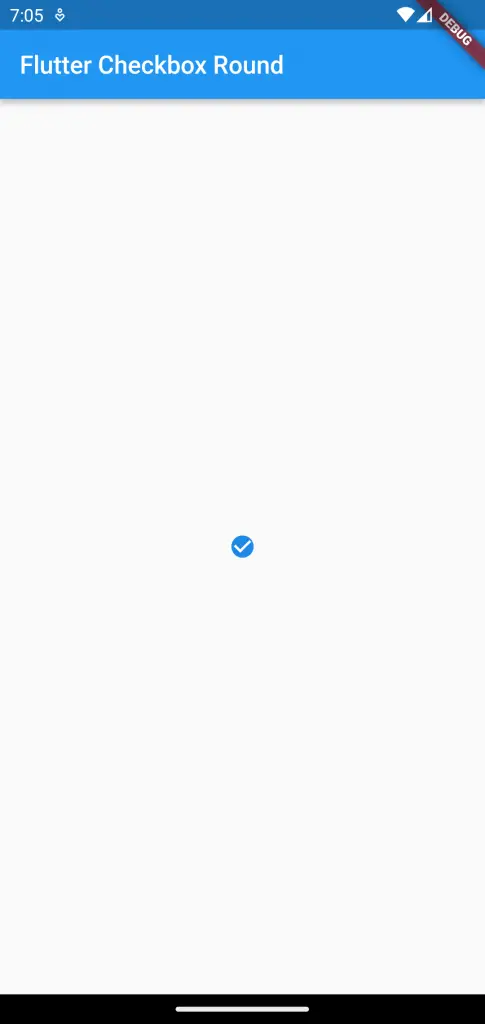
Following is the complete code for reference.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Checkbox Round'),
),
body: const MyStatefulWidget());
}
}
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({super.key});
@override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
bool checkBoxValue = false;
@override
Widget build(BuildContext context) {
return Center(
child: Checkbox(
shape: const CircleBorder(),
value: checkBoxValue,
onChanged: (bool? newValue) {
setState(() {
checkBoxValue = newValue!;
});
},
),
);
}
}
That’s how you create a round CheckBox in Flutter.