How to Use Flex in Flutter Column
In this blog post, we’re focusing on the flex property in Flutter Columns. The use of flex can give you fine-grained control over how widgets behave within a Column
. So, let’s not waste any time and dive right in!
What is Flex?
Before diving into the examples, let’s clarify what flex is. Flex in Flutter is a way to specify how much space a widget will occupy within a flex layout like a Column
. It helps to divide the available space among widgets based on their flex factor.
Flex within a Column
Creating layouts that look good on multiple screen sizes is a common challenge in app development. Flex comes to the rescue when you need dynamic layouts that adjust according to the available space.
Basic Usage
For starters, let’s look at a simple example:
Column(
children: [
Flexible(
flex: 2,
child: Container(
color: Colors.red,
),
),
Flexible(
flex: 1,
child: Container(
color: Colors.green,
),
),
],
)
Code Explanation
- Column Widget: The
Column
is the parent that holds the children vertically. - Flexible Widget: We wrap the children inside
Flexible
widgets. This allows the children to flex their sizes relative to their parent. - Flex Factor: The flex property helps allocate the available space. In this example, the red container takes twice as much vertical space as the green one.
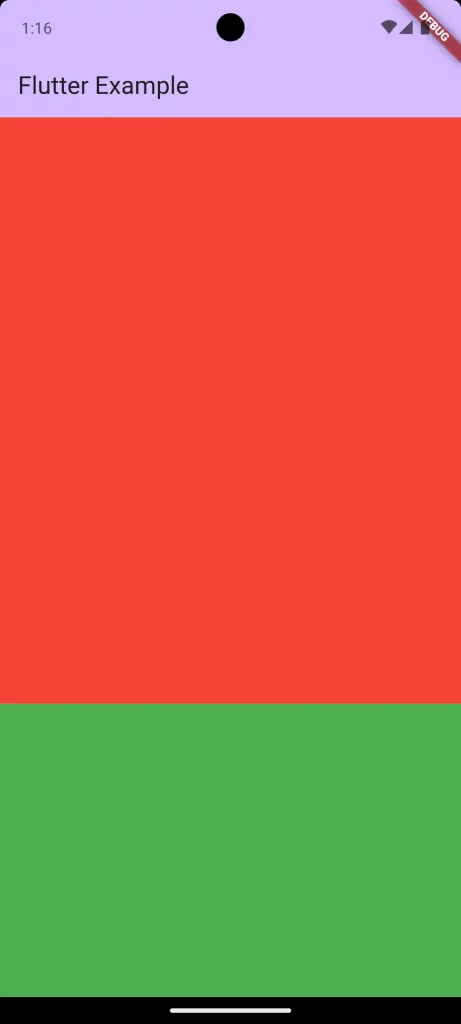
Following is the complete code for reference.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
colorScheme: ColorScheme.fromSeed(seedColor: Colors.deepPurple),
useMaterial3: true,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
backgroundColor: Theme.of(context).colorScheme.inversePrimary,
title: const Text(
'Flutter Example',
),
),
body: Column(
children: [
Flexible(
flex: 2,
child: Container(
color: Colors.red,
),
),
Flexible(
flex: 1,
child: Container(
color: Colors.green,
),
),
],
));
}
}
When to Use Basic Flex
This approach works great when you have a couple of widgets that need to share available space in a predefined ratio.
Advanced Flex Example
For more complex layouts where you may want to insert space between widgets or have more control over widget sizes, using Spacer
and Expanded
widgets can be very useful.
The Code
Column(
children: [
Expanded(
flex: 1,
child: Container(
color: Colors.blue,
),
),
const Spacer(flex: 1),
Expanded(
flex: 2,
child: Container(
color: Colors.orange,
),
),
],
)
Code Explanation
- Expanded Widget: This widget makes the child occupy all available space. If a flex factor is not provided, it defaults to 1.
- Spacer Widget: It’s like an invisible
Container
that can take up a set amount of space. Here, theSpacer
occupies as much vertical space as the blue container. - Combining Widgets: This example shows how to combine
Spacer
andExpanded
widgets to create more complex layouts. The orange container takes twice the vertical space compared to the blue container or the spacer.
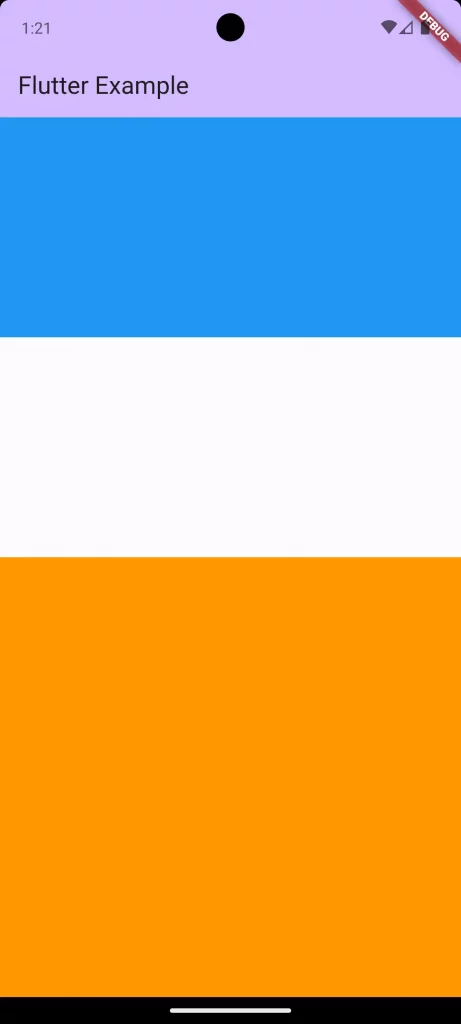
When to Use Advanced Flex
This approach is great when you want to manage both the sizes of the widgets and the spaces between them.
The flex property in a Column
allows for robust control over your layout in Flutter. Whether you are going for a simple design using Flexible
, or need advanced control using Expanded
and Spacer
, understanding flex can be your best friend in UI design.