How to Get Flutter Column Height and Width Using GlobalKey
In this blog post, we’re zeroing in on an advanced technique to find out the height and width of a Column
widget in Flutter: using GlobalKey
. If you’re looking to get these dimensions to use elsewhere in your app, this method is perfect for you.
GlobalKey for Advanced Measurements
When you need to access the dimensions of a widget like a Column
to use in different parts of your code, GlobalKey
becomes an invaluable tool.
The Complete Code
Here’s how to implement it:
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
colorScheme: ColorScheme.fromSeed(seedColor: Colors.deepPurple),
useMaterial3: true,
),
home: MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
MyHomePage({super.key});
final GlobalKey columnKey = GlobalKey();
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
backgroundColor: Theme.of(context).colorScheme.inversePrimary,
title: const Text(
'Flutter Example',
),
),
body: Column(
key: columnKey,
children: [
const Text('First Child'),
const Text('Second Child'),
ElevatedButton(
onPressed: () {
final RenderBox renderBox =
columnKey.currentContext!.findRenderObject() as RenderBox;
final columnWidth = renderBox.size.width;
final columnHeight = renderBox.size.height;
ScaffoldMessenger.of(context).showSnackBar(
SnackBar(
content: Text(
'Column Width: $columnWidth, Column Height: $columnHeight'),
),
);
},
child: const Text('Get Dimensions'),
),
],
));
}
}
Code Explanation
- GlobalKey Initialization: We start by creating a
GlobalKey
and assigning it to thekey
property of theColumn
. - RenderBox: After the
Column
is built, we can fetch itsRenderBox
object usingcolumnKey.currentContext!.findRenderObject()
. - Getting Dimensions:
renderBox.size.width
andrenderBox.size.height
provide us with the width and height of theColumn
. - Show Dimensions: We use a
SnackBar
to display these dimensions when the button is pressed.
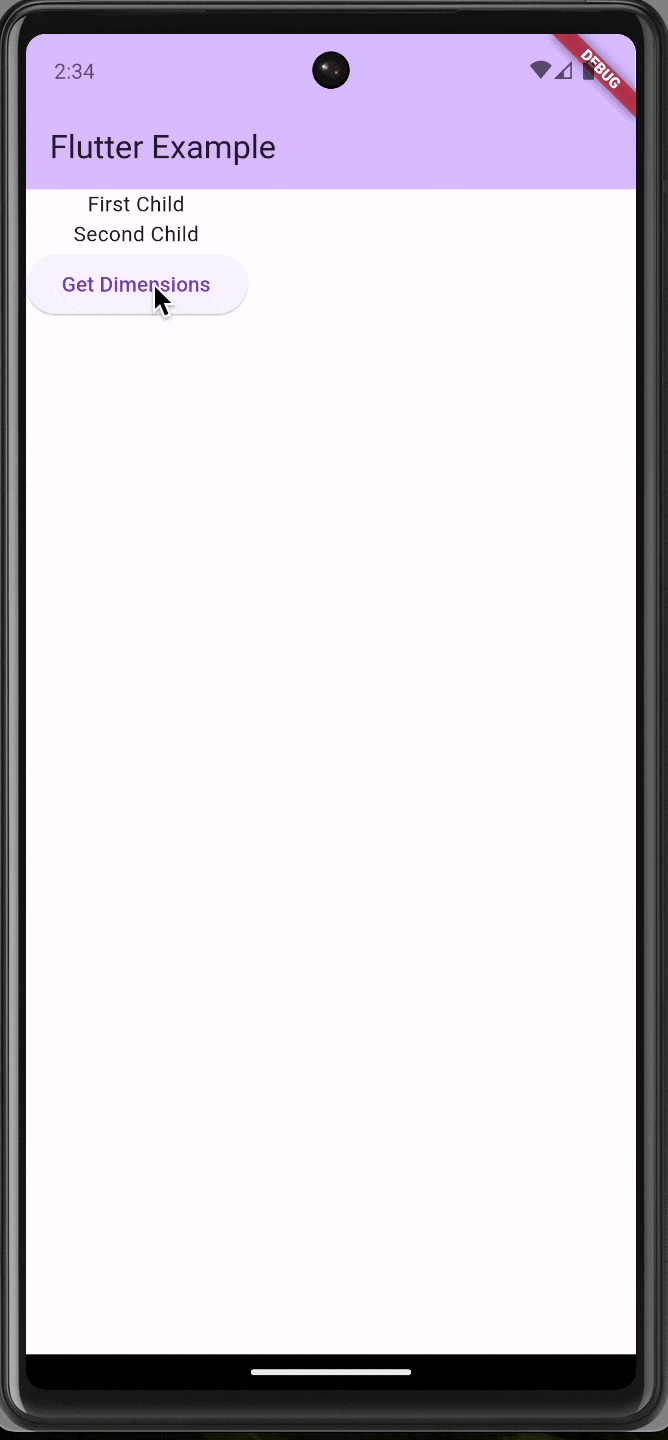
When to Use GlobalKey
The GlobalKey
approach is extremely useful when you need to access or manipulate widget dimensions outside the widget itself, like in a parent widget or different sections of your code.
Using GlobalKey
to determine the dimensions of a Column
in Flutter offers a lot of flexibility, especially when these measurements are needed in multiple places in your app. Hopefully, this guide has made this advanced method easy for you to understand and implement.