How to Manage Flutter Column Item Spacing
In this blog post, we’re focusing on a foundational aspect of Flutter app development: controlling the spacing between items in a Column
widget. Proper item spacing can significantly improve your app’s readability and user experience.
Why Spacing Matters
Before we get into the technical stuff, it’s important to understand why item spacing is crucial. Good spacing enhances readability, allows each element its own visual space, and helps segregate different sections of your app.
Using mainAxisAlignment
The simplest way to manage spacing in a Column
is by using the mainAxisAlignment
property.
The Code
const Column(
mainAxisAlignment: MainAxisAlignment.spaceAround,
children: [
Text('First Child'),
Text('Second Child'),
Text('Third Child'),
],
)
The mainAxisAlignment
property controls how space is distributed along the main axis of the Column
. Setting it to MainAxisAlignment.spaceAround
will distribute the available space evenly around the items.
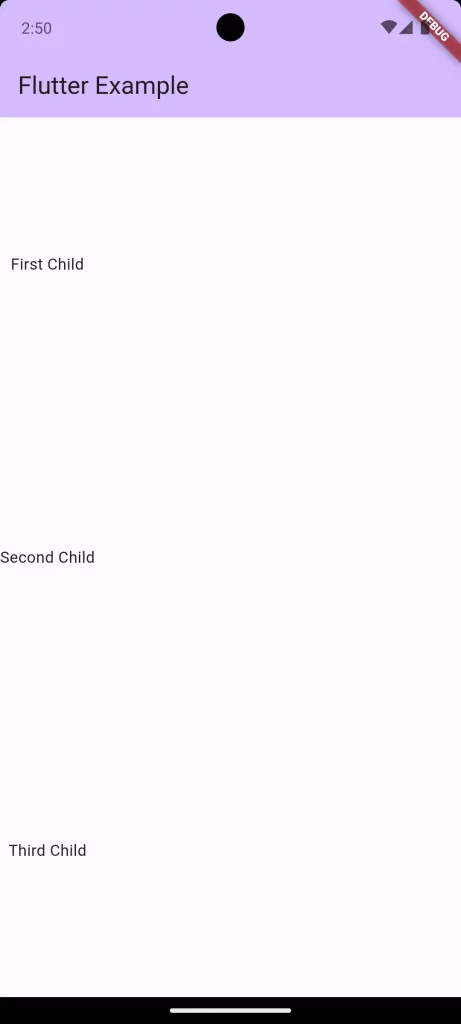
Difference Between Space Types
- spaceAround: Distributes the available space evenly around each child, including before the first and after the last child.
- spaceBetween: Places the available space evenly between the children, but leaves no space at the start or end.
- spaceEvenly: Distributes the available space evenly among all the children, as well as before the first and after the last child.
Following is the complete code for reference.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
colorScheme: ColorScheme.fromSeed(seedColor: Colors.deepPurple),
useMaterial3: true,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
backgroundColor: Theme.of(context).colorScheme.inversePrimary,
title: const Text(
'Flutter Example',
),
),
body: const Column(
mainAxisAlignment: MainAxisAlignment.spaceAround,
children: [
Text('First Child'),
Text('Second Child'),
Text('Third Child'),
],
));
}
}
The Spacer Widget
For more fine-grained control, the Spacer
widget is incredibly useful.
Using Spacer
const Column(
children: [
Text('First Child'),
Spacer(flex: 2),
Text('Second Child'),
Spacer(flex: 1),
Text('Third Child'),
],
)
We inserted Spacer
widgets between the Text
widgets. The flex
property controls the amount of space each Spacer
should occupy relative to the others.
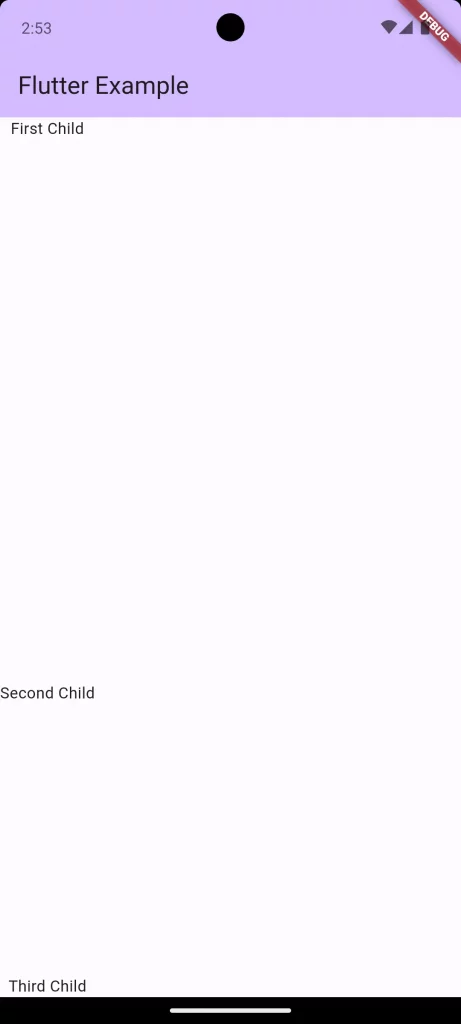
Using Padding for Precise Spacing
Sometimes you might want pixel-perfect spacing. In that case, the Padding
widget can be quite handy.
The Code
const Column(
children: [
Padding(
padding: const EdgeInsets.all(8.0),
child: Text('First Child'),
),
Padding(
padding: const EdgeInsets.all(16.0),
child: Text('Second Child'),
),
],
)
Here, each Text
widget is wrapped in a Padding
widget, allowing you to set the exact padding around each item.
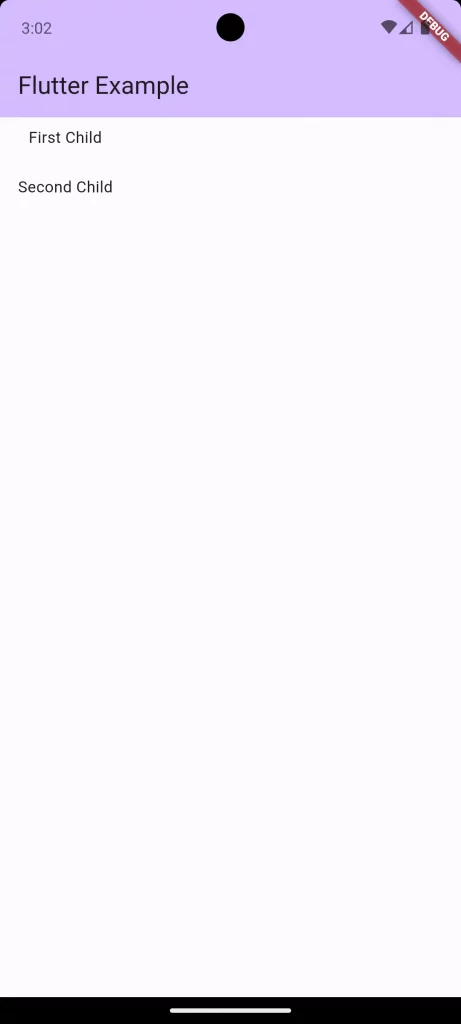
Whether it’s using mainAxisAlignment
, Spacer
, or Padding
, Flutter offers you various tools to achieve perfect spacing in a Column
. Understanding the subtle differences between spaceAround
, spaceBetween
, and spaceEvenly
can help you make more informed choices for your app’s layout.