How to Pass Data from One Screen to Another in Flutter
In this tutorial, let’s explore how to pass data between screens in a Flutter app. Passing data is essential when you want to share information or user input from one screen to another.
Let’s create a simple example with two screens and demonstrate how to send data from the first screen to the second and display it.
We consider the main.dart as the first screen. Then create a file named second_screen.dart for the second screen.
Coming to the code of the first screen, we need to add an interactive widget, such as an ElevatedButton, to the HomeScreen’s body to initiate navigation and pass data. You can place this button inside a Center widget.
Inside the onPressed() callback of the ElevatedButton, use the Navigator.push() method to navigate from the first screen to the second screen. Pass the data to the second screen via its constructor. In this example, we will pass a simple string.
See the code for the first screen, that is, the main.dart file.
import 'package:flutter/material.dart';
import './second_screen.dart';
void main() => runApp(const MyApp());
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
useMaterial3: true,
),
home: const MyHomePage());
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text(
'Flutter Example',
),
),
body: Center(
child: Center(
child: ElevatedButton(
child: const Text('Go to Second Screen with Data'),
onPressed: () {
String data = 'Example data from First Screen';
Navigator.push(
context,
MaterialPageRoute(
builder: (context) => SecondScreen(data: data)),
);
},
),
),
),
);
}
}
Now, when users tap the “Go to Second Screen with Data” button on the first screen, they will be navigated to the second screen along with the data.
Now, we have to display the passed data on the second screen. See the following code for the second screen.
import 'package:flutter/material.dart';
class SecondScreen extends StatelessWidget {
final String data;
const SecondScreen({super.key, required this.data});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Second Screen'),
),
body: Center(
child: Text('Received data: $data'),
),
);
}
}
Here, we declare a final variable called ‘data’ of type String, which will store the data passed to the SecondScreen.
The constructor for the SecondScreen class has two named parameters: key and data. The data parameter is required and marked with the required keyword, which means it must be provided when creating a new SecondScreen instance.
The this.data part assigns the received value to the final variable ‘data’ declared earlier. The Text widget displays the received data.
Following is the output.
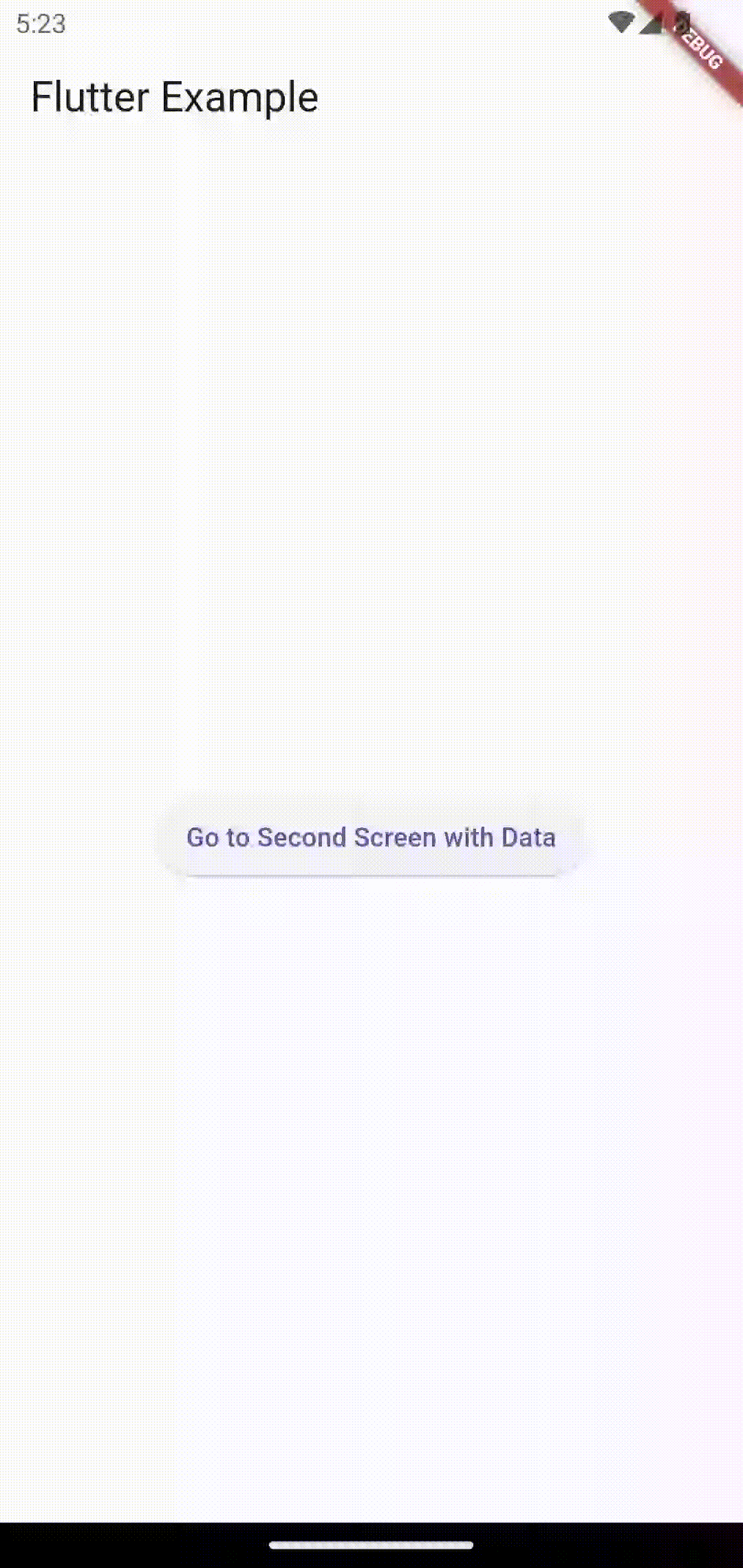
Here, we showed how to pass data between two simple screens in a Flutter app. We used the Navigator.push() method and the widget’s constructor. Additionally, we displayed the received data using a Text widget.