How to Create a Round Image in Flutter
Sometimes, we may want to show images in rounded shapes in our apps. One such example is the profile image of the user. In this Flutter tutorial, let’s check how to add a rounded image in Flutter.
In the previous blog post, how to add an image with rounded corners we used the ClipRRect widget. In this case, we use the ClipOval widget to show the image in a circle shape. We just need to make the Image widget the child of the ClipOval class.
See the code snippet given below.
ClipOval(
child: Image.network(
'https://cdn.pixabay.com/photo/2020/11/04/07/52/pumpkin-5711688_960_720.jpg'),
),
You will get the output with a round image loaded from the internet.
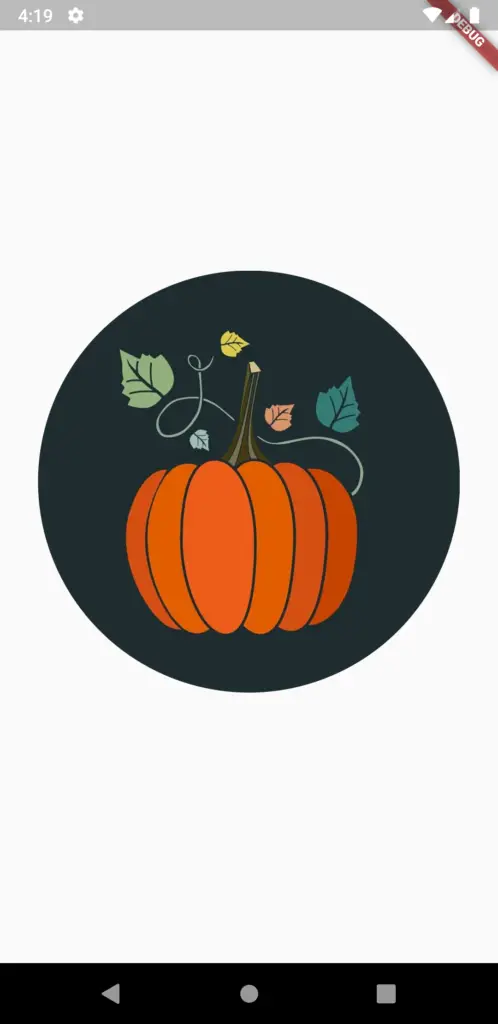
Following is the complete code for the Flutter round image tutorial.
import 'package:flutter/material.dart';
void main() => runApp(App());
class App extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Round Image Example',
home: FlutterExample(),
);
}
}
class FlutterExample extends StatelessWidget {
FlutterExample({Key key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: Padding(
padding: EdgeInsets.all(30),
child:ClipOval(
child: Image.network('https://cdn.pixabay.com/photo/2020/11/04/07/52/pumpkin-5711688_960_720.jpg'),
),),));
}
}
That’s how you add round image in Flutter.