How to change Radio Button Color in Flutter
The Radio widget in Flutter is used to create radio buttons. In this blog post, let’s learn how to change the default color of the radio button in Flutter.
The Radio widget has some useful properties to customize its color. If you just want to change the radio button color when it is active then you should use the activeColor property.
See the following code snippet.
Radio<Animals>(
activeColor: Colors.red,
value: Animals.giraffe,
groupValue: _animal,
onChanged: (Animals? value) {
setState(() {
_animal = value;
});
debugPrint(_animal!.name);
},
)
Then you will get a red color radio button as output.
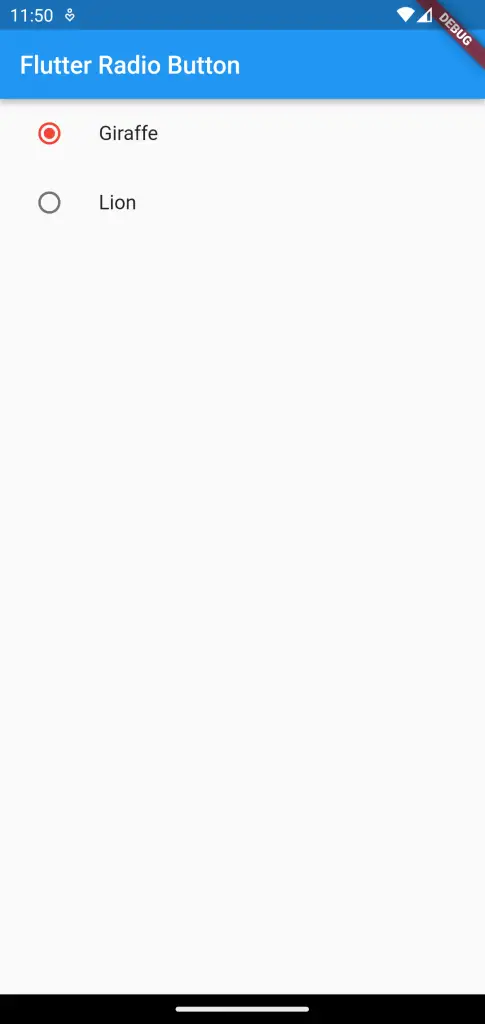
If you want more control over color for different material states then you should choose the fillColor property.
See the following code.
Radio<Animals>(
fillColor: MaterialStateColor.resolveWith(
(Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return Colors.red;
}
return Colors.green;
},
),
value: Animals.giraffe,
groupValue: _animal,
onChanged: (Animals? value) {
setState(() {
_animal = value;
});
debugPrint(_animal!.name);
},
)
Here, the button color is green and it turns red when the button is selected.
The following is the output.
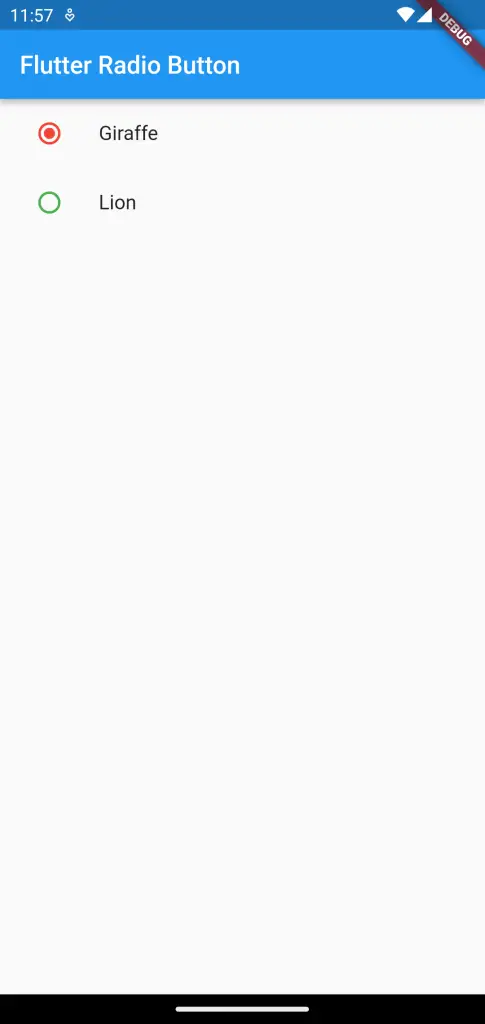
Following is the complete code for reference.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Radio Button'),
),
body: const MyStatefulWidget());
}
}
enum Animals { giraffe, lion }
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({super.key});
@override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
Animals? _animal = Animals.giraffe;
@override
Widget build(BuildContext context) {
return Column(
children: <Widget>[
ListTile(
title: const Text('Giraffe'),
leading: Radio<Animals>(
fillColor: MaterialStateColor.resolveWith(
(Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return Colors.red;
}
return Colors.green;
},
),
value: Animals.giraffe,
groupValue: _animal,
onChanged: (Animals? value) {
setState(() {
_animal = value;
});
debugPrint(_animal!.name);
},
),
),
ListTile(
title: const Text('Lion'),
leading: Radio<Animals>(
fillColor: MaterialStateColor.resolveWith(
(Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return Colors.red;
}
return Colors.green;
},
),
value: Animals.lion,
groupValue: _animal,
onChanged: (Animals? value) {
setState(() {
_animal = value;
});
debugPrint(_animal!.name);
},
),
),
],
);
}
}
That’s how you set the radio button color in Flutter.