How to Apply Padding to Text in Flutter
The importance of padding in mobile app design is very high. The padding helps users to identify different elements of the layout easily. In this short Flutter tutorial, let’s learn how to add padding to Text.
The Padding widget in Flutter helps to apply predefined padding to its children. In the case of Text, you just need to make Text a child of the Padding widget. See the following code snippet for Flutter Text padding.
Padding(
padding: EdgeInsets.all(24.0),
child: Text(
'This is Flutter Text Padding tutorial and how to use it!',
textAlign: TextAlign.center,
style: TextStyle(fontSize: 24, color: Colors.red),
))
Here, the Text is a child of Padding. A padding of 24 is applied to all sides of the Text. The output is given below.
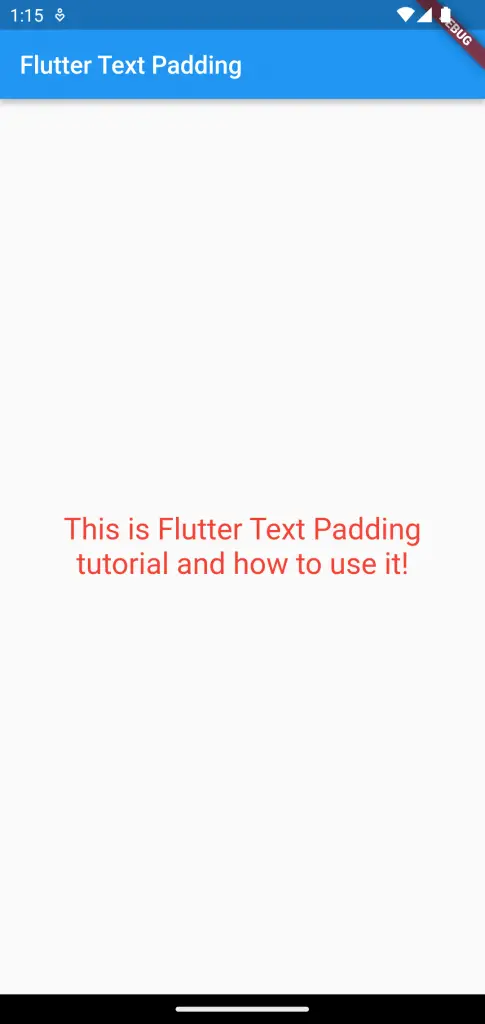
Following is the complete code of this tutorial.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Text Padding'),
),
body: const Center(
child: Padding(
padding: EdgeInsets.all(24.0),
child: Text(
'This is Flutter Text Padding tutorial and how to use it!',
textAlign: TextAlign.center,
style: TextStyle(fontSize: 24, color: Colors.red),
))),
);
}
}
I hope this Flutter Text Padding tutorial is helpful for you.