How to specify Height and Width in Percent with respect to the screen in Flutter
Mobile phone devices are of various sizes and resolutions. Developing a mobile app that looks similar to all these phones is not easy. In such cases, mentioning height and width in percent with respect to the screen can help you.
In Flutter, you can’t really specify some percent of width or height directly. You can make use of MediaQuery class which helps you to obtain metrics of the current screen. MediaQuery.of(context).size.width will give you the current width whereas MediaQuery.of(context).size.height will give you the current height.
Then, multiplying the obtained width or height with fractions will give you height or width with respect to the screen. For example, MediaQuery.of(context).size.width*0.5 will give you 50% width with respect to the screen.
See the Flutter example below where the height of the button is 10% of the height of the screen and the width is 50% of the width of the screen.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Height and Width in Percent Example'),
),
body: Center(
child: SizedBox(
width: MediaQuery.of(context).size.width * 0.5,
height: MediaQuery.of(context).size.height * 0.1,
child: ElevatedButton(
onPressed: () {},
child: const Text('Button in Percent!',
style: TextStyle(fontSize: 20))))));
}
}
Following is the output of this Flutter example.
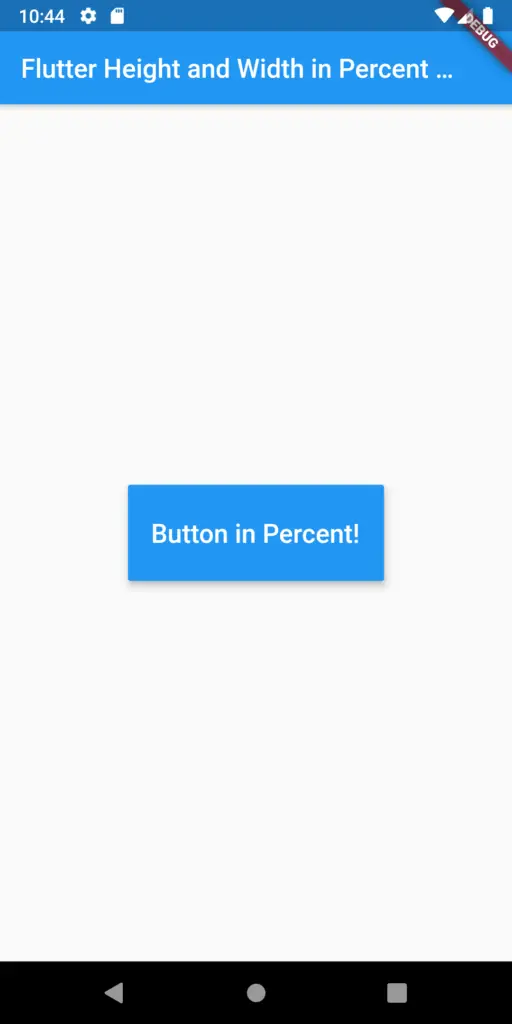
That’s how you define height and width in percent in Flutter.